6 Dec 2015
Select All Elements in the Viewer with View and Data API with JavaScript
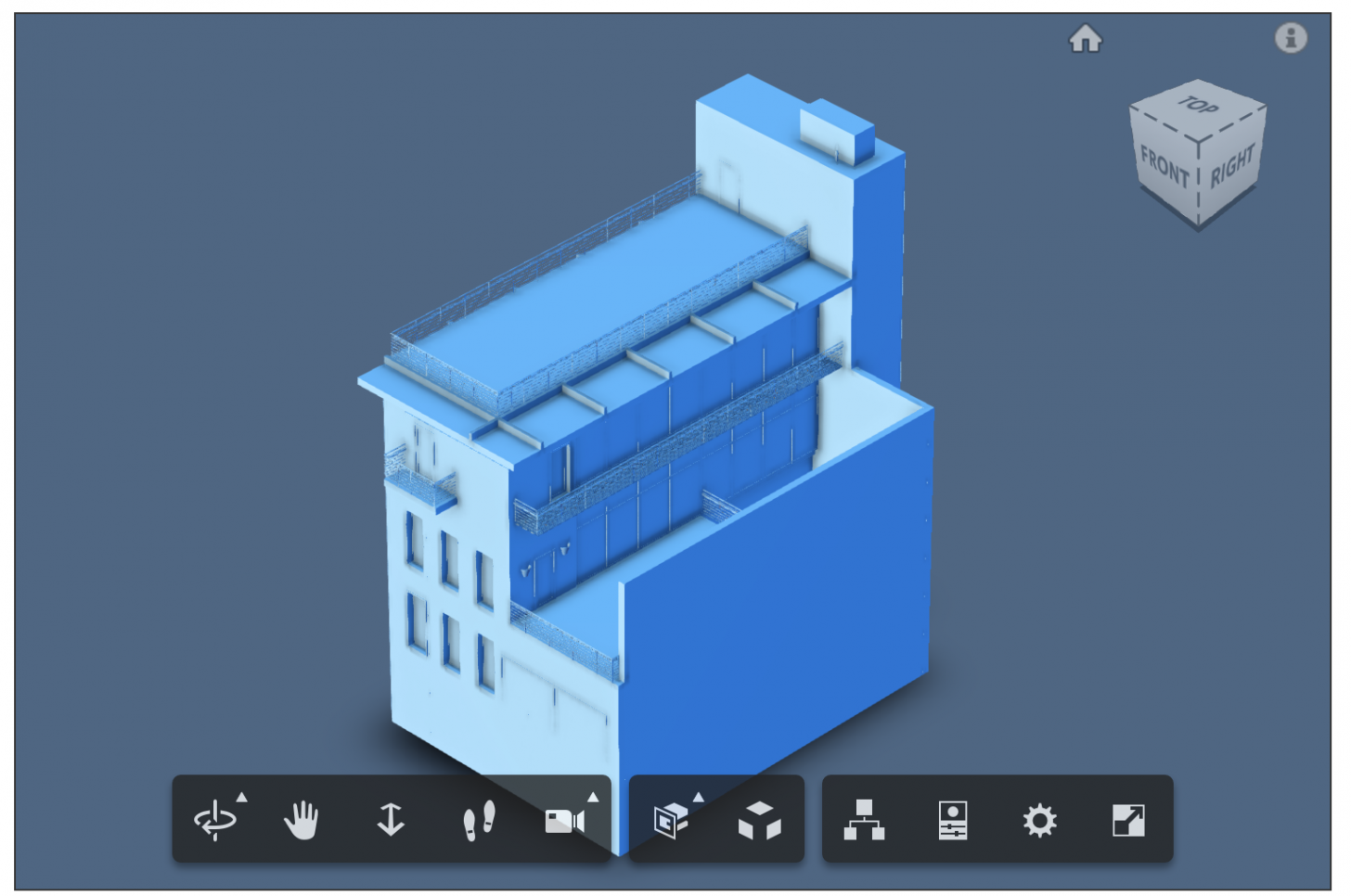
By Shiya Luo
Selecting objects in the viewer requires you to pass in the dbId's of the elements you'd like to select. The select(dbid) function in the Viewer3D class lets you pass in either an integer which is the dbId of the element to select, or an array containing the dbId's of all the elements to select.
Which means to select all the elements, you just need to pass in ALL the dbId's of your model.
The elements are stored as an object tree in the viewer. The getObjectTree(successCallback, errorCallback) function in the Viewer3D class lets you manipulate the model in a tree structure. To get the tree, simply:
var tree; //viewer is your viewer object viewer.getObjectTree(function (objTree) { tree = objTree; });
This saves the object tree as a variable.
Once you got the tree, you want to start with the root:
var root = tree.root;
With the root, you can now do anything with the tree. Each node in the tree contains all the info you can use to trace back to the original model. There are things like
node.children
which gives you all the children of the node, and
node.parent
which gives you all the parents of the node.
The most important one in this case is the
node.dbId
Which gives you the dbId of the element(node).
Now, to select all the elements in the viewer, just write a function that iterate through the tree and push all the dbId's of the nodes into an array. You will pass in this array in the select(dbId) function to select all the elements.
function getAlldbIds (root) { var alldbId = []; if (!root) { return alldbId; } var queue = []; queue.push(root); //push the root into queue while (queue.length > 0) { var node = queue.shift(); // the current node alldbId.push(node.dbId); if (node.children) { // put all the children in the queue too for (var i = 0; i < node.children.length; i++) { queue.push(node.children[i]); } } }; return alldbId; };
This is a very simple breadth-first search algorithm.
To select all the functions, simply pass in all the dbIds:
var allDbIds = getAlldbIds(root); viewer.select(allDbIds);
All the elements are selected in the viewer.
---------------------------
Version 2.5 update:
After version 2.5, the code to get instance tree has changed slightly, see the post: http://adndevblog.typepad.com/cloud_and_mobile/2016/03/breaking-change-in-accessing-model-instance-tree-with-view-data-api.html
Now, to get the instance tree:
var instanceTree = viewer.model.getData().instanceTree; var rootId = this.rootId = instanceTree.getRootId();
With the root id, to get all the ids, use the following code snippet:
function getAlldbIds (rootId) { var alldbId = []; if (!rootId) { return alldbId; } var queue = []; queue.push(rootId); while (queue.length > 0) { var node = queue.shift(); alldbId.push(node); instanceTree.enumNodeChildren(node, function(childrenIds) { queue.push(childrenIds); }); } return alldbId; }