2 Feb 2015
View and Data API Tips: How to set hotkeys for viewer
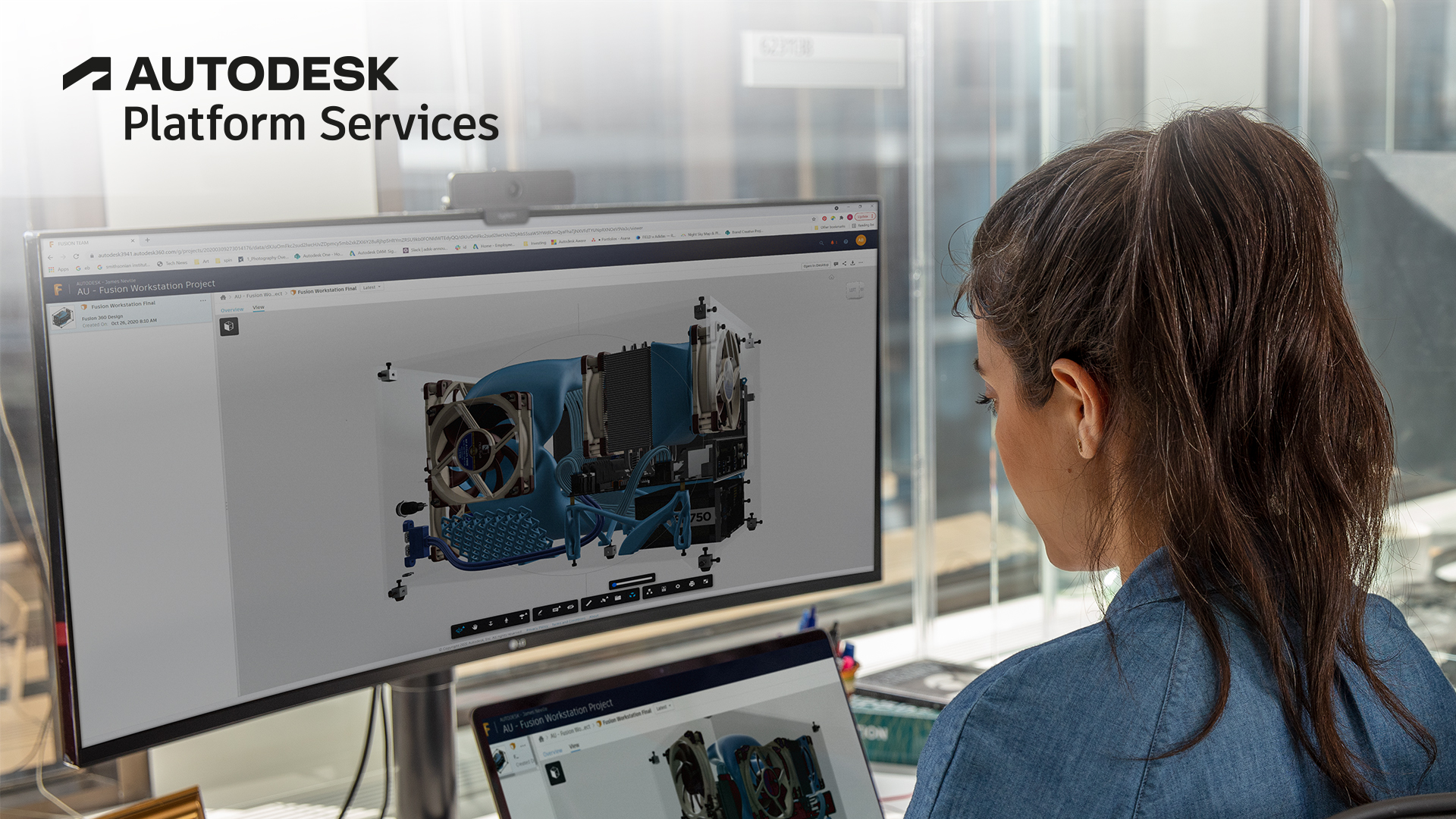
By Daniel Du
In my previous post, I introduced how to make viewer full browser or full screen with view and data API. When playing with it, I am thinking it would be better if we have some hotkeys for these functions, so let’s do it.
In View and Data API, it is simple to register a hotkey, you can do this by hotkey manager. To get the hotkey manager, we can use following code snippet to get the singleton theHotkeyManager, and then we can access the members and methods of it.
//Access the members and methods via the singleton theHotkeyManager. var theHotkeyManager = Autodesk.Viewing.theHotkeyManager;
Next, I define some hotkeys, for example, I define Ctrl + S to trigger my functionality of full screen by calling the setFullScreen(oViewer) in hotkey onReleased event callback. setFullScreen(oViewer) is a function I refactored from last post. It is possible to register multiple hotkeys and organize them into a hotkey set, and then register the hotkey set by calling theHotkeyManager.pushHotkeys().
Here is the code snippet for your reference:
var setFullBrowser = function (viewer) {
var vsmd = new Autodesk.Viewing.ViewerScreenModeDelegate(viewer);
var oldMode = vsmd.getMode();
console.log(oldMode);//kFullScreen, kFullBrowser, kNormal
if (vsmd.isModeSupported(Autodesk.Viewing.Viewer.ScreenMode.kFullBrowser)) {
var newMode = Autodesk.Viewing.Viewer.ScreenMode.kFullBrowser;
vsmd.doScreenModeChange(oldMode, newMode)
}
else {
console.log('Autodesk.Viewing.Viewer.ScreenMode.kFullBrowser not supported');
}
};
var setFullScreen = function (viewer) {
var vsmd = new Autodesk.Viewing.ViewerScreenModeDelegate(viewer);
var oldMode = vsmd.getMode();
console.log(oldMode);//kFullScreen, kFullBrowser, kNormal
if (vsmd.isModeSupported(Autodesk.Viewing.Viewer.ScreenMode.kFullScreen)) {
var newMode = Autodesk.Viewing.Viewer.ScreenMode.kFullScreen;
vsmd.doScreenModeChange(oldMode, newMode)
}
else {
console.log('Autodesk.Viewing.Viewer.ScreenMode.kFullScreen not supported');
}
};
var explode = function (oViewer) {
oViewer.explode(0.7);
}
//Call this in Autodesk.Viewing.GEOMETRY_LOADED_EVENT callback
var configuratorHotKey = function () {
//Access the members and methods via the singleton theHotkeyManager.
var theHotkeyManager = Autodesk.Viewing.theHotkeyManager;
var hotKeyFullScreen = {
keycodes: [theHotkeyManager.KEYCODES.CONTROL,
theHotkeyManager.KEYCODES.s],
onPress: function (hotkeys) {
console.log(hotkeys.toString() + ' pressed');
//has handled
return true;
},
onRelease: function (hotkeys) {
console.log(hotkeys.toString() + ' released');
setFullScreen(oViewer);
//has handled
return true;
}
}
var hotKeyFullBrowser = {
keycodes: [theHotkeyManager.KEYCODES.CONTROL,
theHotkeyManager.KEYCODES.b],
onPress: function (hotkeys) {
console.log(hotkeys.toString() + ' pressed');
//has handled
return true;
},
onRelease: function (hotkeys) {
console.log(hotkeys.toString() + ' released');
setFullBrowser(oViewer);
//has handled
return true;
}
}
//register the hotkey set
var pushOk = theHotkeyManager.pushHotkeys(
'hotkeyFullScreen', //id
[hotKeyFullScreen,
hotKeyFullBrowser],//hotkeys, array
{ tryUntilSuccess: true }//optionsopt
);
if (pushOk) {
console.log('hotkeys - FullScreen - registered.');
}
var hotKeyExplode = {
keycodes: [theHotkeyManager.KEYCODES.CONTROL,
theHotkeyManager.KEYCODES.e],
onPress: function (hotkeys) {
console.log(hotkeys.toString() + ' pressed');
//has handled
return true;
},
onRelease: function (hotkeys) {
console.log(hotkeys.toString() + ' released');
explode(oViewer);
//has handled
return true;
}
}
var pushOk = theHotkeyManager.pushHotkeys(
'explode', //id
[hotKeyExplode],//hotkeys, array
{ tryUntilSuccess: true }//options
);
if (pushOk) {
console.log('hotkeys - explode - registered.');
}
};
Hope this helps! For more code samples, please go to our sample home page: https://developer-autodesk.github.io/