30 Apr 2022
Javascript Wait for Function to Finish
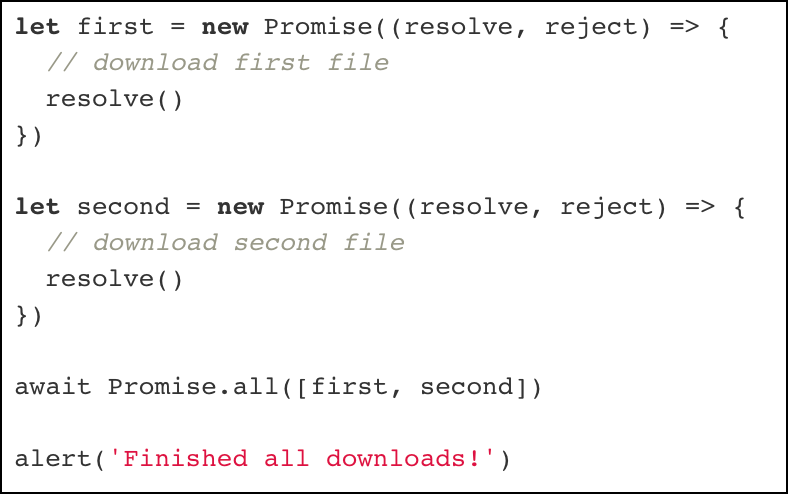
Javascript: Wait for multiple things to finish before continuing
This is not a Forge API related topic, however, I've seen that many people getting started with JavaScript are unaware of Promises and Promise.all() functionality.
How to wait for async calls to finish Javascript
Promises are very useful to make your javascript code readable - instead of passing a callback, and then a callback inside a callback, etc, you can make your code look synchronous by using it along with await:
// note "await" can only be used inside a function flagged as "async"
await new Promise((resolve, reject) => {
// download first file
resolve()
})
await new Promise((resolve, reject) => {
// download second file
resolve()
})
// etc
In order to be more efficient, we can execute the above functions in parallel with the use of Promise.all()
let first = new Promise((resolve, reject) => {
// download first file
resolve()
})
let second = new Promise((resolve, reject) => {
// download second file
resolve()
})
await Promise.all([first, second])
alert('Finished all downloads!')
Just like in case of event handlers, the variables you are using from Promises are accessed later than the declaration of the function, so their values might not be what you expect - unless you know how closures work.