31 Jan 2018
JavaScript on desktop apps (.NET)
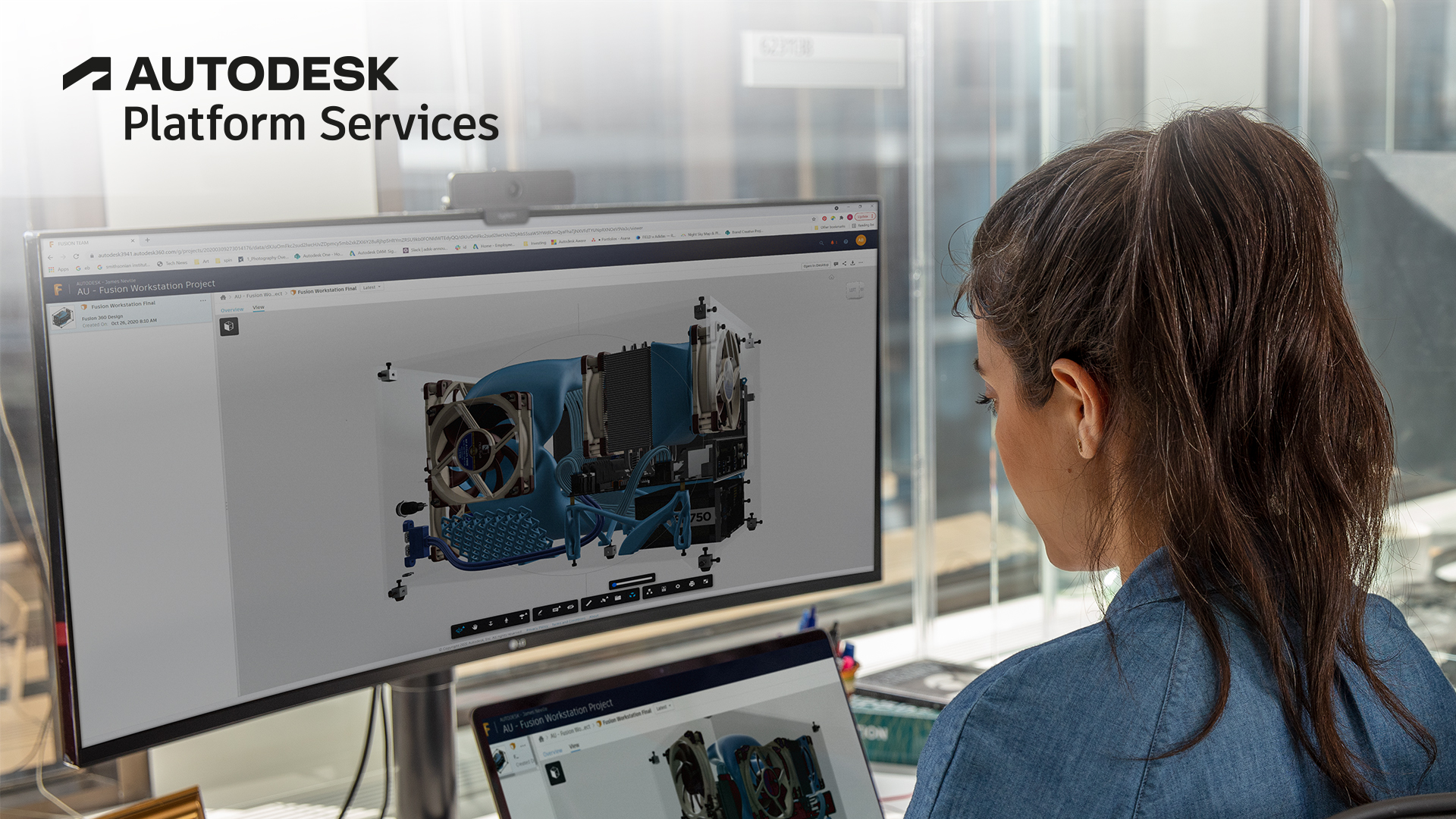
With CefSharp webcontrol we can run a full Chromium browser embedded on our .NET desktop app. That's super powerful! But what about integrating it with our C# .NET routine? Sure, it's possible, just need to think about the scenarios.
First, the JavaScript environment is inside the Chromium and separated from the .NET desktop app, so we need integration points.
1. Run the code and wait for return value: in this case we need to run a JavaScript function and get the result, which is ok in many cases. We basically just need to call .EvaluateScriptAsync() method passing the JavaScript code.
// CefSharp browser
var theScript = @"
(function (){
return LMV_VIEWER_VERSION;
}());
";
browser.EvaluateScriptAsync(theScript)
That's good, but limited as most Viewer methods are asynchronous.
2. Run the code and wait for an output: now let's run the code and wait for some output, let's say the console.log string. In this case our app needs to listed to ConsoleMessage event. Here the browser JavaScript can stringify the JSON and the .NET app can parse it. Simple.
public Form1()
{
InitializeComponent();
// some other code
browser.ConsoleMessage += Browser_ConsoleMessage;
}
private void Browser_ConsoleMessage(object sender, ConsoleMessageEventArgs e)
{
JObject o = JObject.Parse(e.Message);
}
But there is a trick: there are many messages showing on the console, so your app may need to check or only listen during specific times, to avoid mistakes.
As a practical example, let's say our .NET app needs a list of leaf on the model. Based on the code from this post, let's make a small change to stringify the results:
getAllLeafComponents(viewerApp.myCurrentViewer, function (dbIds) {
console.log(JSON.stringify(dbIds);
})
Then, on the console message event, let's use JArray.Parse method. Done. Now the .NET app has the list of integers.