23 Sep 2019
Pack and Go assemblies in Design Automation
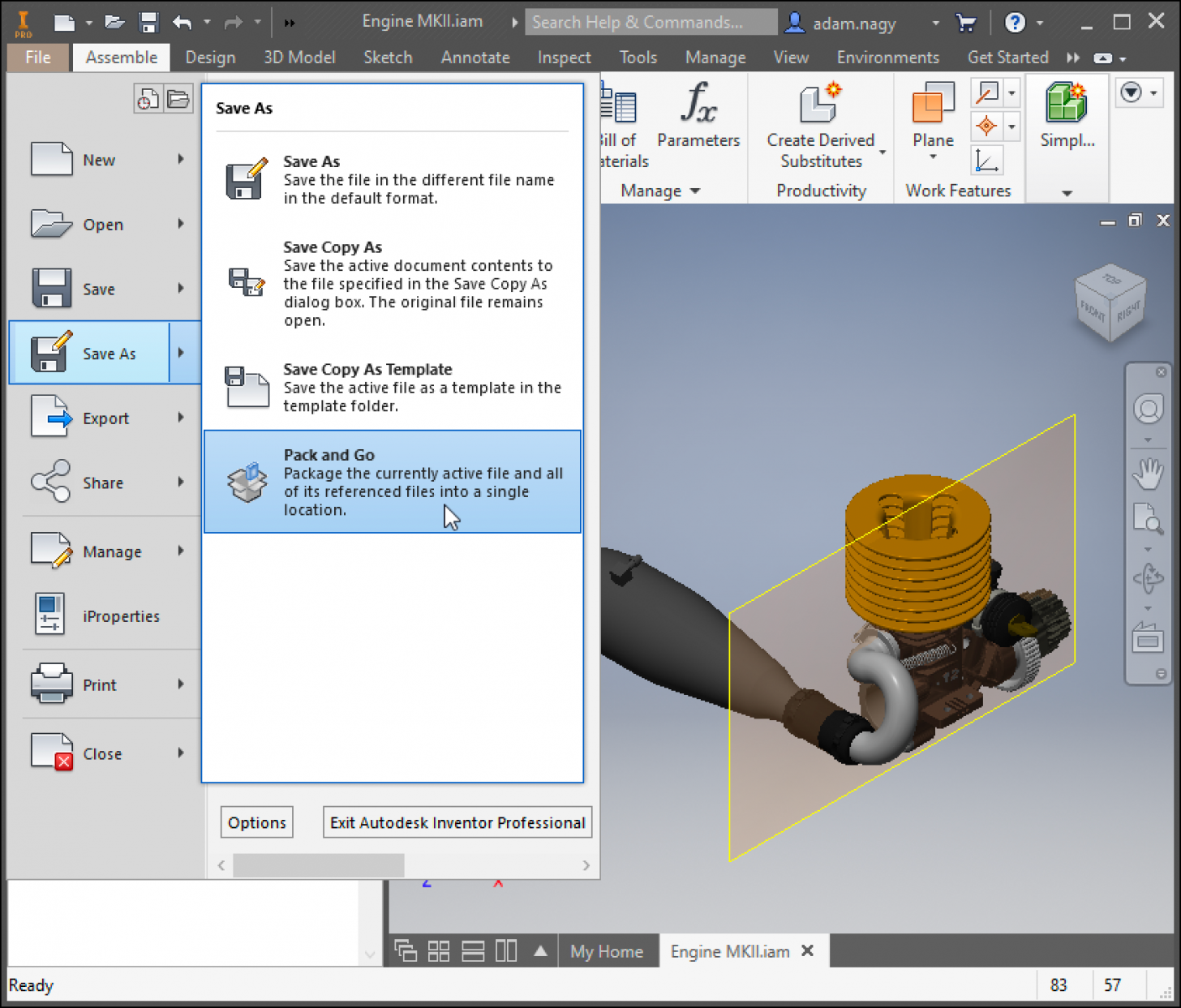
When working with assemblies where the content is scattered across multiple folders - as in case of the sample files provided for Inventor - you can use the "Pack and Go" functionality to make Inventor gather all the necessary files, create a project file (*.ipj) for them and zip them up:
The project file is created for a reason. It helps Inventor find the files it needs. In case of certain functions (like ShrinkWrap apparently) this becomes essential. You can open and activate the project just like in the desktop version of Inventor using the DesignProjectManager object in the Inventor API.
In order to make the AppBundle and Activity work with any input assembly, the parameters in the json file (passed in through the inputJson parameter of the Activity) will also contain the path of both the assembly and the project file - assembyPath and projectPath parameters respectively.
AppBundle code:
public void Run(Document doc)
{
LogTrace("Run()");
string currentDir = System.IO.Directory.GetCurrentDirectory();
LogTrace("Current Dir = " + currentDir);
Dictionary<string, string> parameters = JsonConvert.DeserializeObject<Dictionary<string, string>>(System.IO.File.ReadAllText("params.json"));
foreach (KeyValuePair<string, string> entry in parameters)
{
try
{
LogTrace("Key = {0}, Value = {1}", entry.Key, entry.Value);
}
catch (Exception e)
{
LogTrace("Error with key {0}: {1}", entry.Key, e.Message);
}
}
string assemblyPath = parameters["assemblyPath"];
string projectPath = parameters["projectPath"];
using (new HeartBeat())
{
DesignProject dp = inventorApplication.DesignProjectManager.DesignProjects.AddExisting(System.IO.Path.Combine(currentDir, projectPath));
dp.Activate();
AssemblyDocument asmDoc = inventorApplication.Documents.Open(System.IO.Path.Combine(currentDir, assemblyPath)) as AssemblyDocument;
LogTrace("Opened input assembly file");
AssemblyComponentDefinition compDef = asmDoc.ComponentDefinition;
ObjectCollection ExcludeGroup = inventorApplication.TransientObjects.CreateObjectCollection();
ExcludeGroup.Add(compDef.Occurrences[1]);
PartDocument partDoc = inventorApplication.Documents.Add(DocumentTypeEnum.kPartDocumentObject, "", true) as PartDocument;
LogTrace("Created part document");
PartComponentDefinition partCompDef = partDoc.ComponentDefinition;
ShrinkwrapDefinition SWD = null;
try
{
LogTrace("asmDoc.FullDocumentName = " + asmDoc.FullDocumentName);
SWD = partCompDef.ReferenceComponents.ShrinkwrapComponents.CreateDefinition(asmDoc.FullDocumentName);
LogTrace("After ShrinkwrapComponents.CreateDefinition");
SWD.CreateIndependentBodiesOnFailedBoolean = true;
SWD.DeriveStyle = DerivedComponentStyleEnum.kDeriveAsSingleBodyNoSeams;
SWD.RemoveInternalParts = true;
SWD.AdditionalExcludedOccurrences = ExcludeGroup;
LogTrace("Before ShrinkwrapComponents.Add");
ShrinkwrapComponent SWComp = partCompDef.ReferenceComponents.ShrinkwrapComponents.Add(SWD);
LogTrace("Saving part document");
partDoc.SaveAs(System.IO.Path.Combine(currentDir, "outputFile.ipt"), false);
LogTrace("Saved part document");
}
catch (Exception ex)
{
LogTrace("Error: " + ex.Message);
}
}
}
commandLine parameter for the Activity:
(note that it's missing the part that opens the model, since we'll be doing that from our AppBundle's code)
["$(engine.path)\\InventorCoreConsole.exe /al $(appbundles[<name of appbundle>].path)"]
Parameters to start WorkItem:
{
"inputFile": {
"zip": true,
"localName": "input",
"url": "<input URL>"
},
"inputJson": {
"localName": "params.json",
"url": "data:application/json,{\"assemblyPath\":\"input\\\\EngineSample\\\\Workspaces\\\\Workspace\\\\Assemblies\\\\Engine MKII\\\\Engine MKII.iam\", \"projectPath\":\"input\\\\EngineSample\\\\Engine MKII.ipj\", \"height\":\"16 in\", \"width\":\"10 in\"}"
},
"outputFile": {
"localName": "outputFile.ipt",
"url": "<output URL>",
"verb": "put"
}
}