5 Nov 2024
Introducing the Application Management API Sample Application: A Guide to Automatic Secret Rotation
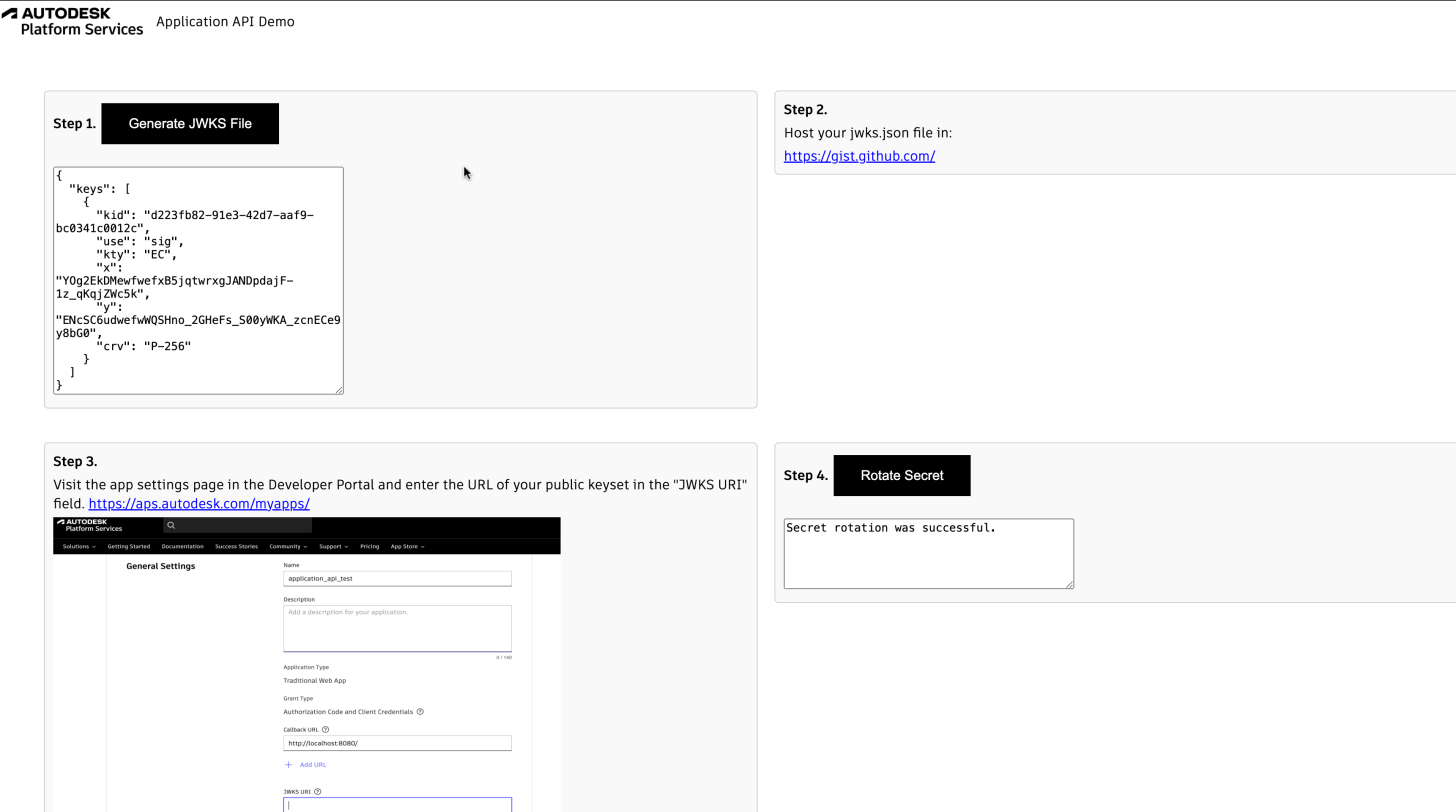
The Autodesk Application Management API sample helps developers securely rotate application secrets with zero downtime. For more details, refer to the sample application. https://github.com/autodesk-platform-services/application-management-api-sample
Why Secret Rotation is Important
Secret rotation is critical for maintaining secure access between services without interruptions. By integrating this process with key management systems like AWS KMS or Azure Key Vault, businesses can enhance security, avoid service disruptions, and ensure compliance with security protocols.
How the API Helps
The sample demonstrates how to rotate secrets programmatically with Node.js using services like AWS Lambda or Azure Functions. Here's a breakdown of the process:
- Creating a Key
The API generates a key pair using the Elliptic Curve algorithm and prepares it for secure use:
export async function createKey() { const keyPair = await jose.generateKeyPair("ES256"); const kid = crypto.randomUUID(); const publicKey = JSON.stringify( { keys: [{ kid, use: "sig", ...(await jose.exportJWK(keyPair.publicKey)) }] }, null, 2 ); const privateKey = await jose.exportPKCS8(keyPair.privateKey); return { publicKey, privateKey }; }
- Committing a New Secret
This part handles the actual update of the secret in Autodesk’s Application API:
export async function commitSecret(newSecret) { const accessToken = await getAccessToken(); const url = `https://developer.api.autodesk.com/applications/v1/clients/${CLIENT_ID}/secret:commit`; const clientAssertion = await sign({ iss: CLIENT_ID, sub: CLIENT_ID, aud: url, }); await axios.post( url, { clientAssertion: clientAssertion, newSecret: newSecret }, { headers: { Authorization: `Bearer ${accessToken}` } } ); }
The code handles creating the new key, preparing the JWT assertion, and securely updating the secret while ensuring uninterrupted service. This approach ensures a seamless secret rotation process for developers managing sensitive information in cloud environments.
For more information and a complete walkthrough, visit the developer guide https://aps.autodesk.com/en/docs/applications/v1/developers_guide/overview/