21 Nov 2019
Fast Track Your Cross Platform React Native Forge App with the Expo SDK - Part One
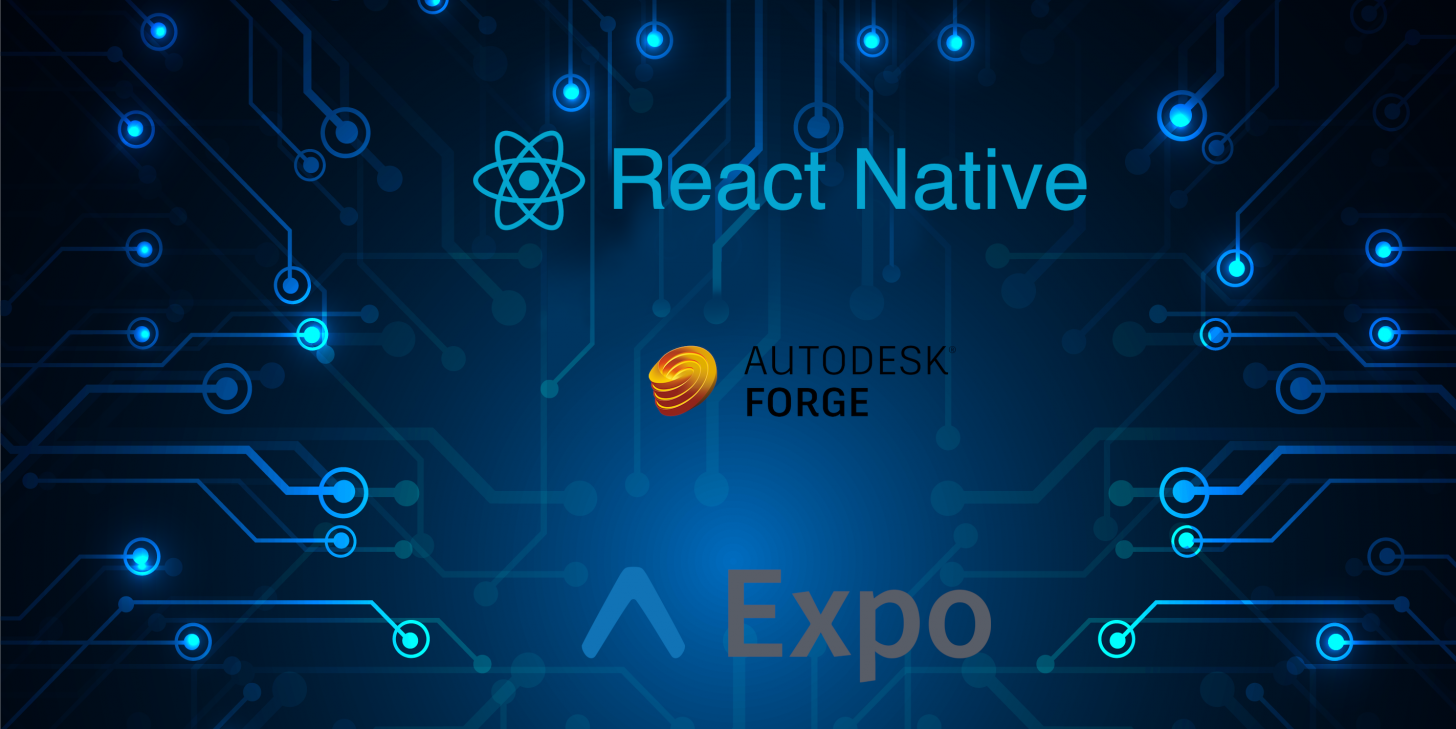
Ever since Forge came to be we've been having inquiries about what'd be the way to go about building Forge apps with React Native - among many options available the Expo SDK and tools have been gaining traction for the last couple of years. Today we are going to explore some its great features that suit our common needs for a Forge Viewer app as well as the developer experience on which it prides itself so much.
What's Expo?
Expo is an open source, freely available SDK, which is used to build your cross platform react native apps. Expo also provides online playground for react native apps, in which you can write code for your apps and check in both iOS and Android devices. And yes the beauty of it really is when you don’t have to have a MAC or iOS system to develop for iOS, just try Expo!
Getting Started
You can follow the official guide here to install the Expo CLI globally which is arguably the best way to start but be careful with one pitfall - if you initialize your project would attempt to install a dependency from Github and that might cause the NPM to hang. To work around this - once the project has been finished scaffolding let's interrupt the subsequent NPM install:
expo init myProject // ctrl-c at the NPM install phase
Edit the dependencies to use the published versions and then install the dependencies manually with "npm i", for example here's how I set up my "package.json":
"dependencies": {
"expo": "^35.0.1",
"react": "^16.8.3",
"react-dom": "^16.8.3",
"react-native": "^0.59.10"
And this is how our sample project looks like:
Setting Up Viewer
As of now Forge Viewer is still available only as JavaScript and renders on WebGL - so we will still need to fallback to the H5 stack by rendering inside a WebView.
Like we went through one of the greatest features about Expo, among many of them, is to get you started in no time and here we go to straight away - simply tuck in a WebView to the main app component and set up a HTML for the Viewer app as usual:
render() {
//...
return (
//...
<WebView
source={{uri:'http://path/to/viewer/html/'}}
javaScriptEnabled={true}
/>
//...
);
}
And to load the HTML as an app asset simply try:
<WebView
source={{html: contentString, baseUrl: url}}
//...
Be sure to specify a base URL otherwise features depending on the presence of domains like cookies wont’t work ...
Loading Models as Local Assets
Unfortunately Viewer does not support the File protocol so we will need to leverage the two way communication APIs to pass model resources to the WebView - here we will need ServiceWorker to intercept Viewer requests (similiar topics discussed here and here) and be sure to pass the binary in as data(base64) string:
//...
import { Asset } from 'expo-asset'
export default class component extends Component {
//...
onMessage = (data) => {
Asset.loadAsync(require('./path/to/models/'+data.url)).then(()=>{
this.webview.postMessage({url:data.url, contents: btoa(Asset.fromModule(require('./path/to/models/'+data.url)))});
})
}
//...
<WebView
onMessage={this.onMessage}
//...
See here for a sample project to get you started and load the model as bundled assets - we will address relevant topics in greater details in the second part of this series.
Running
Simply start the debug and bundling server with:
npm start // equivalent to 'expo start'
// or
expo start --android/ios // to run a specific platform only
Your browser will then navigate to the debug console:
In addition to the tranditional simulator options, you can then simply scan the QR code and run the app in the Expo client app available on both iOS and Android - here's the output on my Android Nougat:
And don't forget their mind blowing online playground - Expo Snack! I've got a very basic Viewer app there and you will need to run it in your device/simulator unfortunately as WebGL is disabled online (probably not if you have a paid Apptize account).
Debugging
It's a real cinch to debug with Expo. Apart from wonderful debugging experience offered by the Expo CLI (see details here) their client apps offer great features like stack printing, hot reloading etc:
On the WebView front, apart from the traditional remote browser debugger, try a remote debugging tool like Vorlon.js, Cypress or Tenon! Take Vorlon for example all it takes is to load its client script in your HTML:
<script src="http://hostname:1337/vorlon.js"></script>
Then enjoy the interactive console, element/listener inspection, event tracking, performance analysis and many more:
Building and Publishing
You can build to iOS, Android and web platforms and that'd require an account with Expo. Highly recommend to delegate petty tasks like version and credentials/keystore management, and even distribution etc to the Expo tools- see here for details.
Plus there's tons of features available in its configuration that's way to broad to capture in this tiny little blog of mine!
Alright that's all we have time for today - let us know if you have any specific questions and see here to reach out to us. Take care and until next time! ;)