24 Sep 2019
Pass path to Design Automation
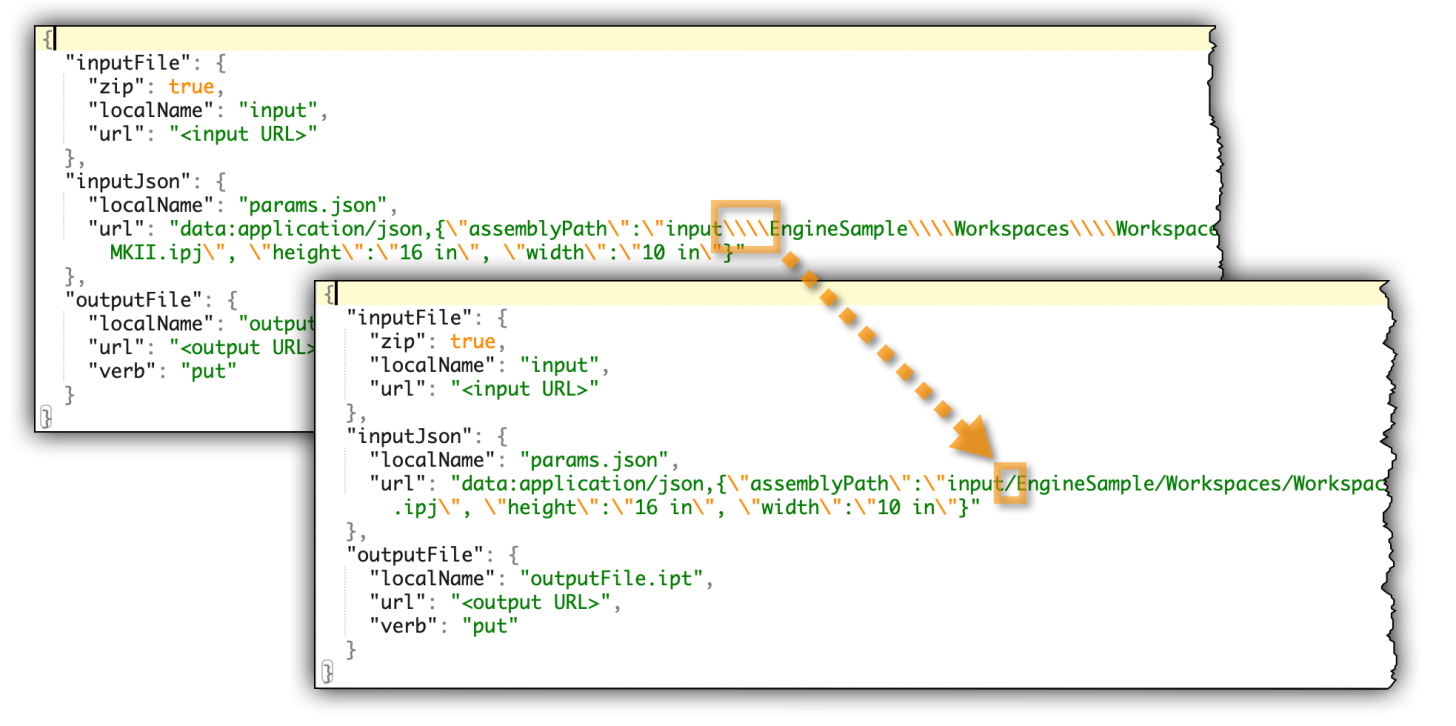
Depending on what your AppBundle and Activity is designed to do, it may need a relative path for some of the files.
On Windows the backslash '\' is used as the directory separator character, which, unfortunately, is also used as an escape character in strings.
This means that depending on how many processing steps a string goes through, you might have to keep doubling the backslashes to get a single backslash at the end of the road.
E.g. after some trial and error, I found that I needed to add 4 of them so that things work correctly inside my AppBundle's code when providing the string inside the UI of https://da-manager.autodesk.io/ - see WorkItem arguments used in Pack and Go assemblies in Design Automation
One simple way to avoid this is to use forward slash '/' characters instead for folder separation. .NET functions can work with that too, however, as I found out, it breaks the file resolution part provided by DesignProjectManager object in the Inventor API, when the project file is loaded with a path using forward slashes.
You can easily convert the forward slash characters back to the default one by using System.IO.Path.GetFullPath() - you could also do a character replace (i.e. string.replace('/', '\')) in the string, but I think GetFullPath() is nicer ?
So instead of providing this for the WorkItem:
"inputJson": {
"localName": "params.json",
"url": "data:application/json,{\"assemblyPath\":\"input\\\\EngineSample\\\\Workspaces\\\\Workspace\\\\Assemblies\\\\Engine MKII\\\\Engine MKII.iam\", \"projectPath\":\"input\\\\EngineSample\\\\Engine MKII.ipj\", \"height\":\"16 in\", \"width\":\"10 in\"}"
},
I can use this:
"inputJson": {
"localName": "params.json",
"url": "data:application/json,{\"assemblyPath\":\"input/EngineSample/Workspaces/Workspace/Assemblies/Engine MKII/Engine MKII.iam\", \"projectPath\":\"input/EngineSample/Engine MKII.ipj\", \"height\":\"16 in\", \"width\":\"10 in\"}"
},
And use this code to combine the relative path with the current directory:
DesignProject dp = inventorApplication.DesignProjectManager.DesignProjects.AddExisting(
System.IO.Path.GetFullPath(System.IO.Path.Combine(currentDir, projectPath)));
dp.Activate();
Note: System.IO.Path.Combine() does not modify forward slashes to backslashes since both are accepted characters for folder separation.
So System.IO.Path.Combine("C:\temp\folder", "myfolder/subfolder") will simply produce "C:\temp\folder\myfolder/subfolder"