14 Aug 2023
Load specific sheet and get its thumbnail
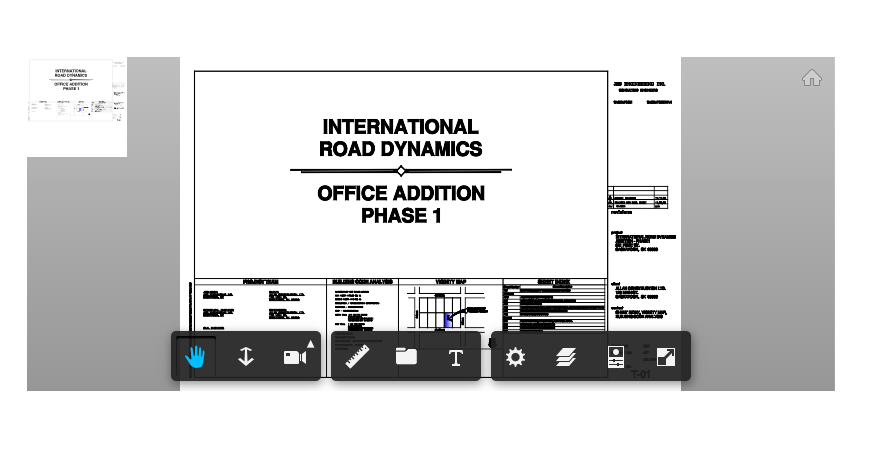
Loading a sheet
There are 3 different extensions that deal with presenting 2D sheets: LeafletLoader (PDF translated to SVF), PDFLoader (PDF loaded directly), and F2DLoader (RVT, DWG, etc translated to SVF)
In the case of LeafletLoader and F2DLoader you first load the document using Autodesk.Viewing.Document.load (just like in the case of 3D models), then search for the view you want to load and open it using viewer.loadDocumentNode
Autodesk.Viewing.Document.load(documentId, (doc => {
var sheets = doc.getRoot().search(
{
type: "geometry",
role: "2d",
},
true
);
viewer.loadDocumentNode(doc, sheets[0], {})
}));
In the case of PDFLoader, there is no bubble generated for the PDF (we're loading it directly without translation), so you need to use viewer.loadModel.
That will generate info about all the pages which then you can find using viewer.model.getDocumentNode().getRootNode()
viewer.loadModel("/sample.pdf", { page: 1 }, model => {
var sheets = model.getDocumentNode().getRootNode().search(
{
type: "geometry",
role: "2d",
},
true
);
console.log(sheets);
});
Once you know how many pages the document has you can just pass in the index of the specific one you want load by setting the page option also used in the above code.
If you want to move back and forth between pages, you can also take advantage of the DocumentBrowser extension talked about here: Switch between sheets
Get sheet thumbnail
The DocumentBrowser extension handles this as well, so you can just debug into its source code to see how it does it.
It's using a function called Autodesk.Viewing.Thumbnails.getUrlForBubbleNode and you can just pass in any valid item of the sheet array used in either of the above shown functions.
If you have an <img> element on your page you can just assign the generated data to its src property:
viewer.loadModel("/sample.pdf", { page: 1 }, model => {
var sheets = model.getDocumentNode().getRootNode().search(
{
type: "geometry",
role: "2d",
},
true
);
console.log(sheets);
Autodesk.Viewing.Thumbnails.getUrlForBubbleNode(sheets[0]).then(src => {
document.getElementById("thumbnail").src = src;
})
});
The main picture of this article shows the result.