22 Apr 2021
Switch between sheets
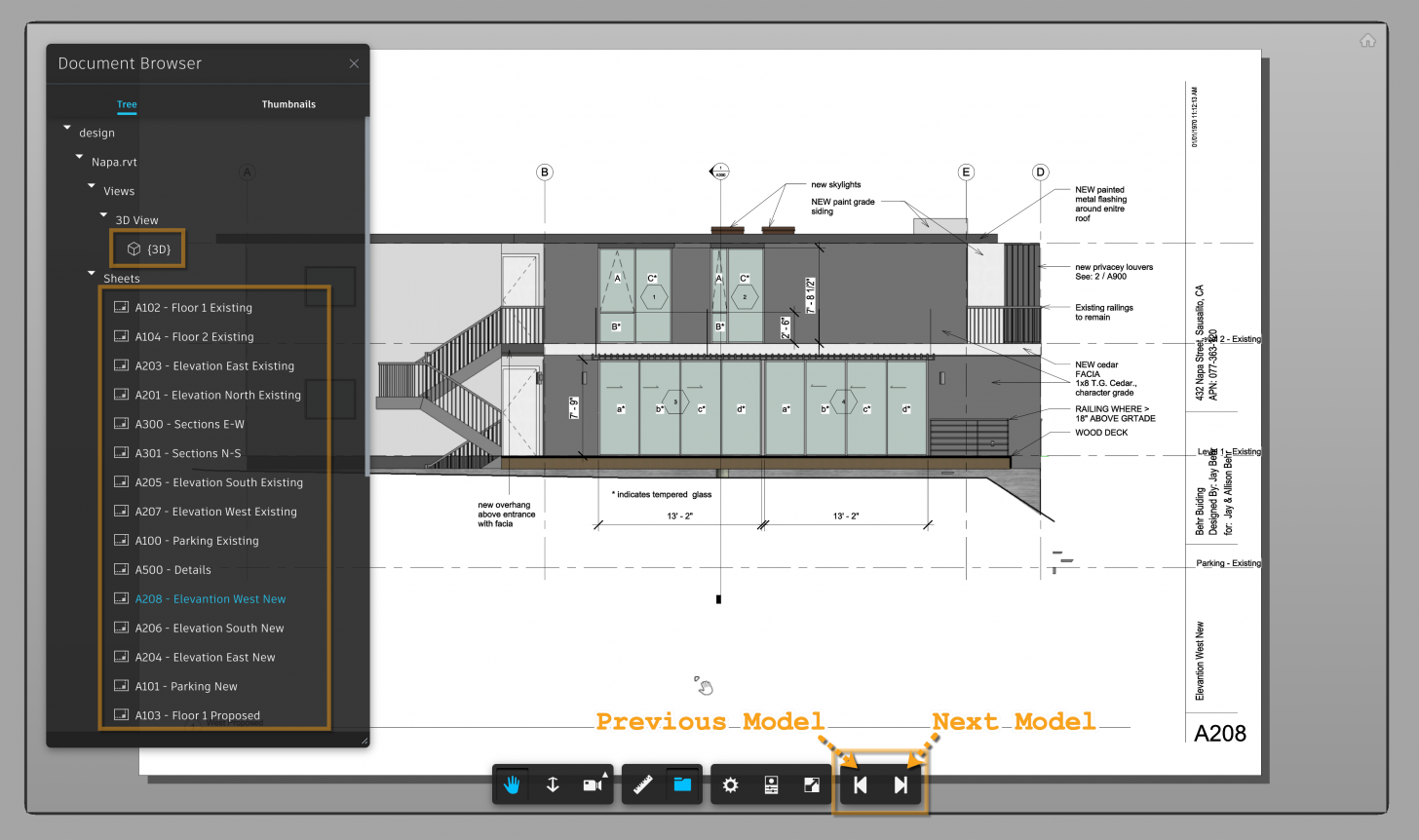
Certain file types can have multiple models in them. These tend to be 2d sheets as in the case of Revit projects (*.rvt), PDF files, Inventor drawings (*.idw), etc
There is no direct built-in way to show the available models and enable the user to switch between the sheets, however, there is an extension for it that you can load into the Viewer. It's called "Autodesk.DocumentBrowser"
Apart from providing a Panel that lists all the available sheets and their thumbnails, it also exposes two functions to go back and forth between those sheets: loadNextModel() / loadPrevModel()
In this sample, we created an extension that will provide toolbar buttons that can navigate to the previous and next model in the document. This extension and the "Autodesk.DocumentBrowser" extension are both loaded using the { extensions: [] } option of the GuiViewer3D() constructor.
<html>
<head>
<meta
name="viewport"
content="width=device-width, minimum-scale=1.0, initial-scale=1, user-scalable=no"
/>
<meta charset="utf-8" />
<!-- The Viewer CSS -->
<link
rel="stylesheet"
href="https://developer.api.autodesk.com/modelderivative/v2/viewers/7.*/style.min.css"
type="text/css"
/>
<link
rel="stylesheet"
href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.11.2/css/all.min.css"
/>
<!-- Developer CSS -->
<style>
body {
margin: 0;
}
#MyConytainerDiv {
width: 100%;
height: 100%;
position: relative;
}
#MyViewerDiv {
width: 100%;
height: 100%;
margin: 0;
background-color: #f0f8ff;
}
</style>
<title>Showing A360 Shared files</title>
</head>
<body>
<!-- The Viewer will be instantiated here -->
<div id="MyConytainerDiv">
<div id="MyViewerDiv"></div>
</div>
<!-- The Viewer JS -->
<script src="https://developer.api.autodesk.com/modelderivative/v2/viewers/7.*/viewer3D.js"></script>
<!-- jQuery -->
<script
src="https://code.jquery.com/jquery-3.2.1.min.js"
integrity="sha256-hwg4gsxgFZhOsEEamdOYGBf13FyQuiTwlAQgxVSNgt4="
crossorigin="anonymous"
></script>
<!-- Developer JS -->
<script>
// this is the iframe URL that shows up when sharing a model embed on a page
var embedURLfromA360 =
"https://myhub.autodesk360.com/ue29c89b7/shares/public/SH7f1edQT22b515c761e81af7c91890bcea5?mode=embed"; // Revit file (A360/Forge/Napa.rvt)
var viewer;
function getURN(onURNCallback) {
$.get({
url: embedURLfromA360
.replace("public", "metadata")
.replace("mode=embed", ""),
dataType: "json",
success: function (metadata) {
if (onURNCallback) {
let urn = btoa(metadata.success.body.urn)
.replace("/", "_")
.replace("=", "");
onURNCallback(urn);
}
},
});
}
function getForgeToken(onTokenCallback) {
$.post({
url: embedURLfromA360
.replace("public", "sign")
.replace("mode=embed", "oauth2=true"),
data: "{}",
success: function (oauth) {
if (onTokenCallback)
onTokenCallback(oauth.accessToken, oauth.validitySeconds);
},
});
}
getURN(function (urn) {
var options = {
env: "AutodeskProduction",
getAccessToken: getForgeToken,
};
var documentId = "urn:" + urn;
Autodesk.Viewing.Initializer(options, function onInitialized() {
Autodesk.Viewing.Document.load(documentId, onDocumentLoadSuccess);
});
});
/**
* Autodesk.Viewing.Document.load() success callback.
* Proceeds with model initialization.
*/
function onDocumentLoadSuccess(doc) {
// A document contains references to 3D and 2D viewables.
var items = doc.getRoot().search(
{
type: "geometry",
role: "2d",
},
true
);
if (items.length === 0) {
console.error("Document contains no 2d sheets.");
return;
}
var viewerDiv = document.getElementById("MyViewerDiv");
viewer = new Autodesk.Viewing.GuiViewer3D(viewerDiv, { extensions: [
"Autodesk.DocumentBrowser", "MyExtension"
]});
viewer.start();
viewer.loadDocumentNode(doc, items[1], {})
}
class MyExtension extends Autodesk.Viewing.Extension {
onToolbarCreated() {
let group = viewer.toolbar.getControl("modelToolbar");
if (!group) {
group = new Autodesk.Viewing.UI.ControlGroup("modelToolbar");
viewer.toolbar.addControl(group);
}
// Add a new button to the toolbar group
let button = new Autodesk.Viewing.UI.Button("prevButton");
button.icon.classList.add("fa", "fa-step-backward");
button.onClick = () => {
let ext = viewer.getExtension("Autodesk.DocumentBrowser");
// Workaround to make things work with local PDFs
// Not needed from v7.98 on
ext.geometries = ext.rootNode.search({type: "geometry", role: "2d"});
ext.loadPrevModel();
};
button.setToolTip("Previous Model");
group.addControl(button);
button = new Autodesk.Viewing.UI.Button("nextButton");
button.icon.classList.add("fa", "fa-step-forward");
button.onClick = () => {
let ext = viewer.getExtension("Autodesk.DocumentBrowser");
// Workaround to make things work with local PDFs
// Not needed from v7.98 on
ext.geometries = ext.rootNode.search({type: "geometry", role: "2d"});
ext.loadNextModel();
};
button.setToolTip("Next Model");
group.addControl(button);
}
}
Autodesk.Viewing.theExtensionManager.registerExtension('MyExtension', MyExtension);
</script>
</body>
</html>