5 Dec 2023
Check the version of a Revit file using Automation API
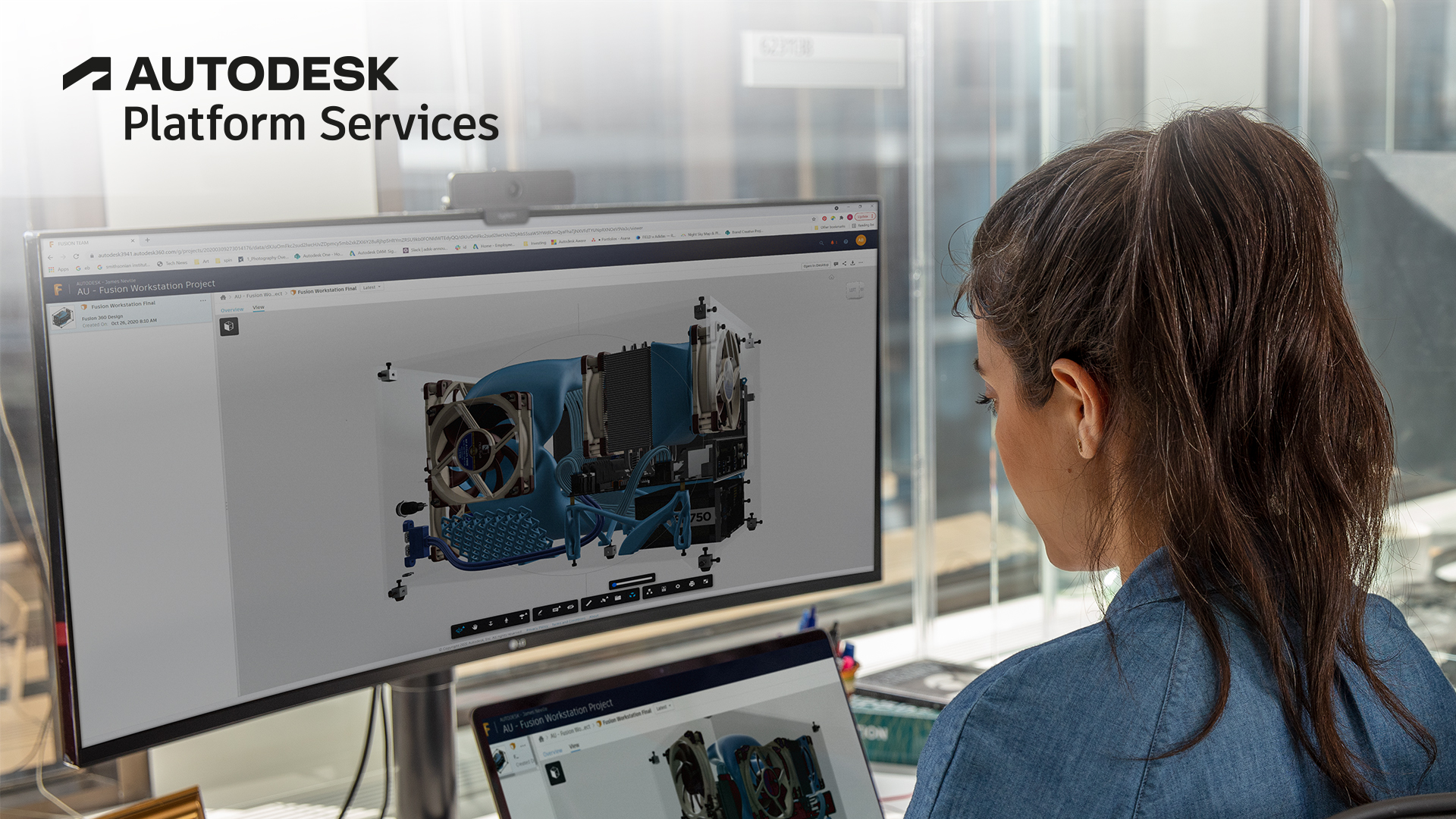
In a previous blog post, we discussed two methods to determine the version of a Revit file in https://aps.autodesk.com/blog/check-version-revit-file-hosted-cloud. One uses the Model Derivative API, and the other is a workaround that checks a few encoded bytes of the Revit file.
However, there may be situations where these approaches are not suitable for your needs. For instance, the Revit file may not be processed through the Model Derivative API, or you may not want to wait for the Model Derivative translation just to obtain the file version. But don't worry, we have a solution for you!
Revit API has a helper class called BasicFileInfo, which can extract basic information such as Revit version, worksharing status, username, central path, and other details about an RVT & RFA file without fully opening it, according to the current API design.
In addition, the BasicFileInfo can support extracting information from RVT & RFA files saved in older Revit versions, which means we can use the BasicFileInfo of Revit 2024 API to extract basic file information from RVT & RFA files saved with Revit 2024, Revit 2023, Revit 2022 and so on, according to the API documentation. Here is a code snippet showing how it works:
var filename = @"./rac_basic_sample_project.rvt";
var basicFileInfo = BasicFileInfo.Extract(filename);
JsonConvert.SerializeObject( basicFileInfo );
// {
// "AllLocalChangesSavedToCentral": false,
// "CentralPath": "",
// "Format": "2020",
// "IsCentral": false,
// "IsCreatedLocal": false,
// "IsInProgress": false,
// "IsLocal": false,
// "IsSavedInCurrentVersion": false,
// "IsSavedInLaterVersion": false,
// "IsValidObject": true,
// "IsWorkshared": false,
// "LanguageWhenSaved": 0,
// "LatestCentralEpisodeGUID": "14da91f7-bb8f-46ac-a0d0-3d8917d007d1",
// "LatestCentralVersion": 15,
// "Username": ""
// }
By this,
We can utilize the Revit BasicFileInfo API to develop a Revit add-in that can be executed on Automation using the latest Revit engine version (currently Revit 2024). This add-in will help us extract the Revit version from RVT and RFA files.
Here is a code sample: https://github.com/yiskang/DA4R-RevitBasicFileInfoExtract, and the result would look like the example below:
[
{
"filename": "Commercial_Sample_Project.rvt",
"allLocalChangesSavedToCentral": false,
"centralPath": "",
"format": "2020",
"isCentral": false,
"isCreatedLocal": false,
"isInProgress": false,
"isLocal": false,
"isSavedInCurrentVersion": false,
"isSavedInLaterVersion": false,
"isValidObject": true,
"isWorkshared": false,
"languageWhenSaved": 0,
"latestCentralEpisodeGUID": "da4dd9b0-b1aa-4d09-9097-99fb28d0d153",
"latestCentralVersion": 11,
"username": ""
},
{
"filename": "rst_basic_sample_project.rvt",
"allLocalChangesSavedToCentral": false,
"centralPath": "",
"format": "2021",
"isCentral": false,
"isCreatedLocal": false,
"isInProgress": false,
"isLocal": false,
"isSavedInCurrentVersion": false,
"isSavedInLaterVersion": false,
"isValidObject": true,
"isWorkshared": false,
"languageWhenSaved": 0,
"latestCentralEpisodeGUID": "c3556c99-d20b-4f92-bdc0-67ffdf7be96e",
"latestCentralVersion": 48,
"username": ""
},
{
"filename": "サンプル意匠.rvt",
"allLocalChangesSavedToCentral": false,
"centralPath": "",
"format": "2024",
"isCentral": false,
"isCreatedLocal": false,
"isInProgress": false,
"isLocal": false,
"isSavedInCurrentVersion": true,
"isSavedInLaterVersion": false,
"isValidObject": true,
"isWorkshared": false,
"languageWhenSaved": 8,
"latestCentralEpisodeGUID": "2ab934ef-56c5-4a10-80c7-87ec865046d6",
"latestCentralVersion": 92,
"username": ""
},
{
"filename": "rst_basic_sample_family.rfa",
"allLocalChangesSavedToCentral": false,
"centralPath": "",
"format": "2022",
"isCentral": false,
"isCreatedLocal": false,
"isInProgress": false,
"isLocal": false,
"isSavedInCurrentVersion": false,
"isSavedInLaterVersion": false,
"isValidObject": true,
"isWorkshared": false,
"languageWhenSaved": 0,
"latestCentralEpisodeGUID": "8c42dcab-f657-41a4-a9ee-243c996cc358",
"latestCentralVersion": 59,
"username": ""
}
]
Afterward,
To make the workflow smoother and more efficient while using the Revit Automation API, we can use the following steps, which ensure that the correct version of the Revit engine is selected to help prevent work-item failures and help to avoid waiting for Revit to upgrade the file during opening.
- Submit a work-item of Revit BasicFileInfo API discussed above to extract the Revit file version for the input.
- Choose and submit your actual/main work-item with the matched Revit engine version, based on the returned result from Step 1.