3 Aug 2017
Using Autodesk.Viewing.MarkupsCore extension
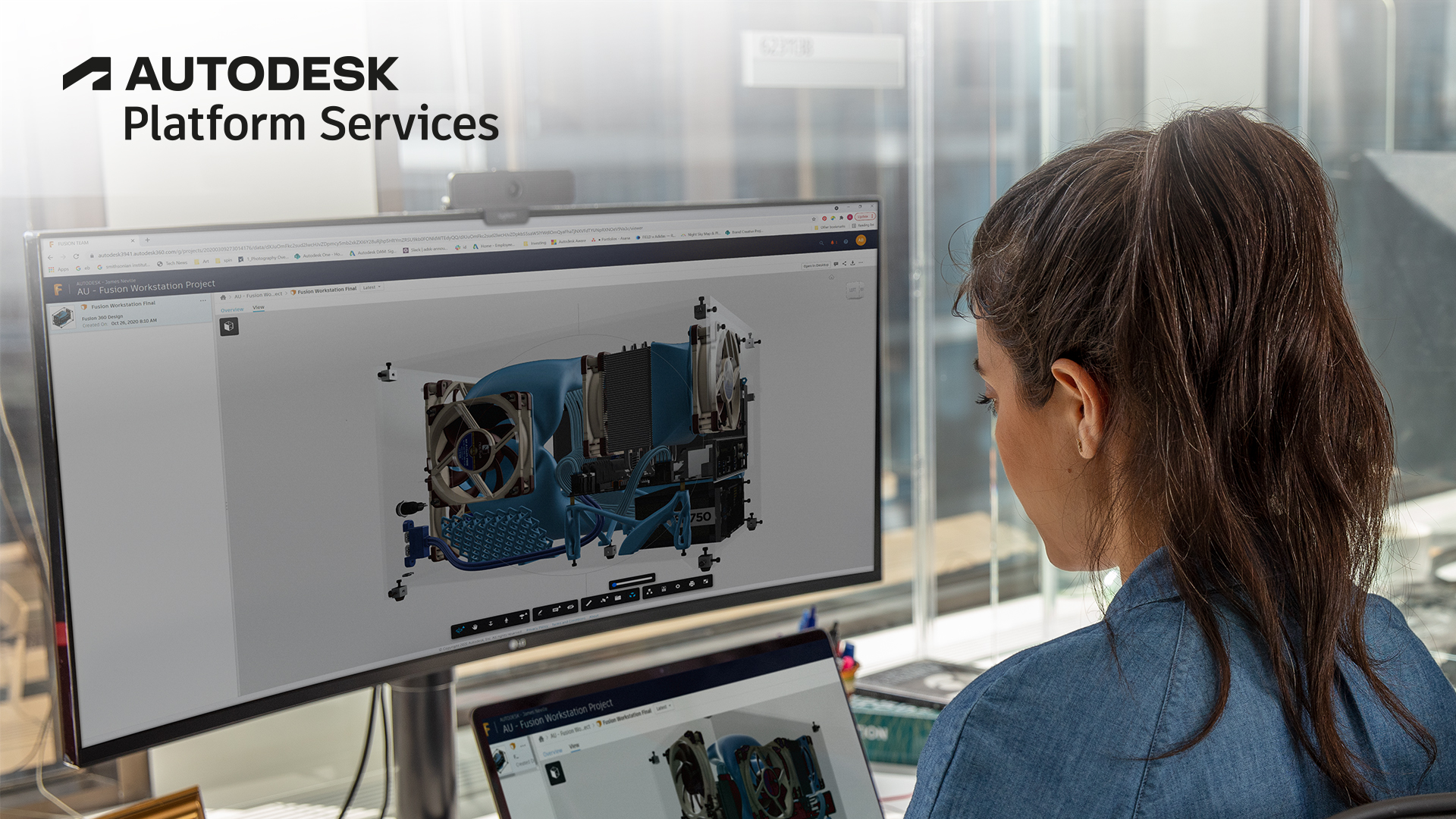
Let's experiment with Autodesk.Viewing.MarkupsCore on Viewer 2.16! Thanks to Eason Kang for helping debug it.
First, make sure your code is using this version:
<link rel="stylesheet" href="https://developer.api.autodesk.com/viewingservice/v1/viewers/style.min.css?v=v2.16" type="text/css" />
<script src="https://developer.api.autodesk.com/viewingservice/v1/viewers/three.min.js"></script>
<script src="https://developer.api.autodesk.com/viewingservice/v1/viewers/viewer3D.min.js?v=v2.16"></script>
Do you know you can write quick testing code directly in the browser console? That's handy! Let's load the extension with Promise:
var markup;
NOP_VIEWER.loadExtension('Autodesk.Viewing.MarkupsCore').then(function(markupsExt){
markup = markupsExt;
});
Here is how it looks like on the console:
Tip: in a page with a Viewer, we can access it with NOP_VIEWER, easier. If we just type a variable name, like markup that we just declared, it will output its value.
Now let's add a Cloud revision:
markup.enterEditMode();
var cloud = new Autodesk.Viewing.Extensions.Markups.Core.EditModeCloud(markup)
markup.changeEditMode(cloud)
And the result should be:
We can change the edit more to different options:
EditModeArrow
EditModeCircle
EditModeCloud
EditModeFreehand
EditModeHighlight
EditModePen
EditModePolycloud
EditModePolyline
EditModeRectangle
EditModeText
Tip: you can see the above list by typing Autodesk.Viewing.Extensions.Markups.Core on the console.
Now we need to persist this information. Once we finish the edit mode we cannot edit the markups again, it is converted into a SVG output. Also, we need to keep a record of the view state.
// markups we just created as a string
var markupsPersist = markup.generateData()
// current view state (zoom, direction, sections)
var viewerStatePersist = markup.viewer.getState()
// finish edit of markup
markup.leaveEditMode()
// hide markups (and restore Viewer tools)
markup.hide()
Next is up to your implementation: submit the markupsPersist and viewerStatePersist to your server-side and store on your database. Later you can restore with:
// restore the view to the state where the markups were created
markup.viewer.restoreState(viewerStatePersist)
// show markups
markup.show();
// show the markups on a layer
markup.loadMarkups(markupsPersist, "layerName")