6 May 2024
Three-Legged token with Python and Flask
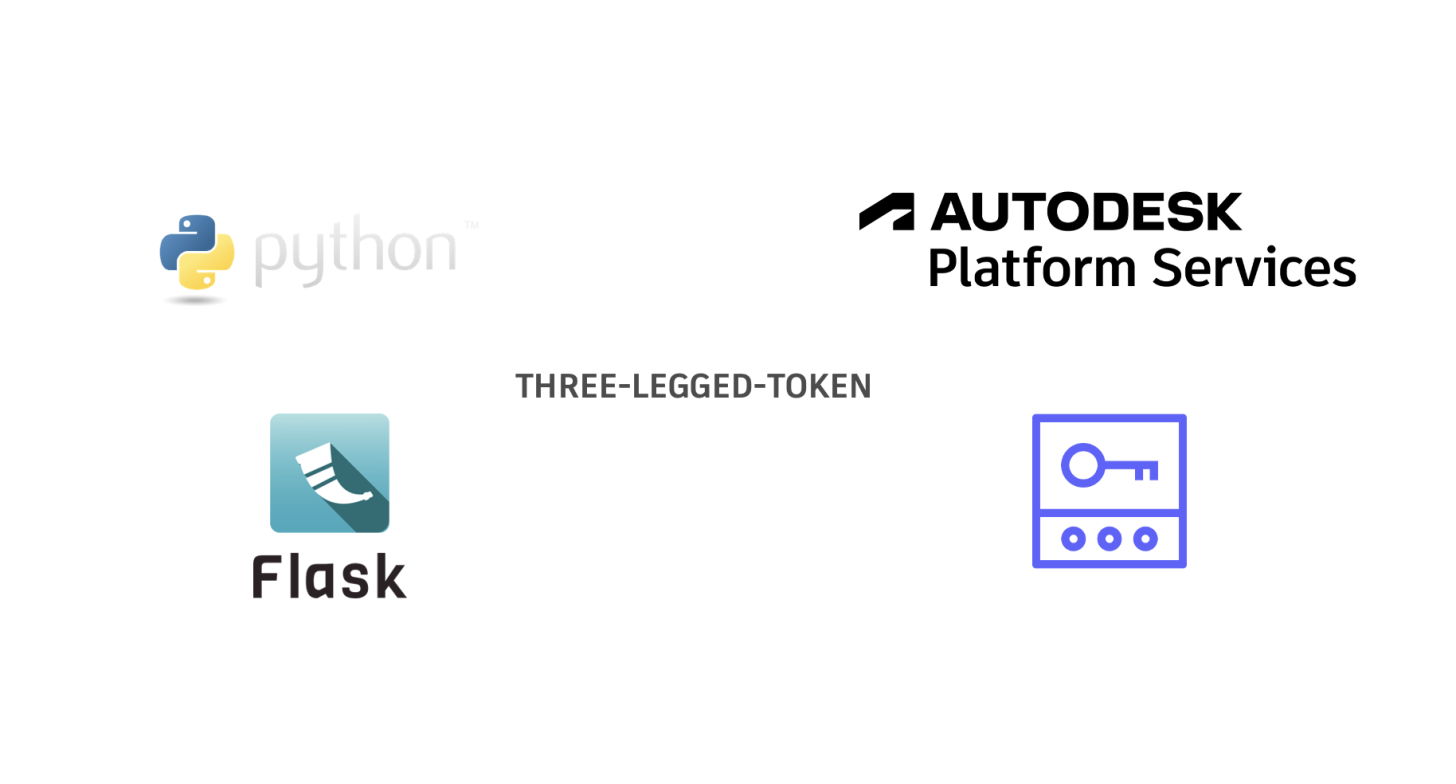
Introduction
You may need to obtain three-legged tokens in your first steps using APS with Python, and we currently don't have an official SDK to address that (yet).
To save you the trouble of handling everything independently from scratch we've put together this minimal sample to help you quickly obtain a three-legged token using Python with a Flask server.
How it works?
First, you'll need to install Flask in your environment.
You'll also need to specify the proper CALLBACK URL in your APS app accordingly (more details in our tutorial).
Then, you can use the code below to obtain the token.
from flask import Flask, request, redirect
import requests
import os
from dotenv import load_dotenv
app = Flask(__name__)
# Load configuration variables from .env
load_dotenv()
@app.route('/')
def authenticate():
return redirect(f"https://developer.api.autodesk.com/authentication/v2/authorize?response_type=code&client_id={os.getenv('CLIENT_ID')}&redirect_uri={os.getenv('CALLBACK_URL')}&scope={os.getenv('SCOPES')}")
@app.route('/api/auth/callback', methods=['POST','GET'])
def callback():
# Get credential code
code = request.args.get('code')
payload = f"grant_type=authorization_code&code={code}&client_id={os.getenv('CLIENT_ID')}&client_secret={os.getenv('CLIENT_SECRET')}&redirect_uri={os.getenv('CALLBACK_URL')}"
tokenUrl = "https://developer.api.autodesk.com/authentication/v2/token"
headers = {
"Content-Type": "application/x-www-form-urlencoded"
}
resp = requests.request("POST", tokenUrl, data=payload, headers=headers)
respJson = resp.json()
# Return success response
return f"{respJson}", 200
if __name__ == '__main__':
app.run(debug=True, port=8080)
The code basically redirects the user to sign in on Autodesk Authorization Server, then exchanges the credential code received for a token, as described in our documentation.
You can find the complete source code below: