15 May 2024
Migrating to the new APS .NET SDK
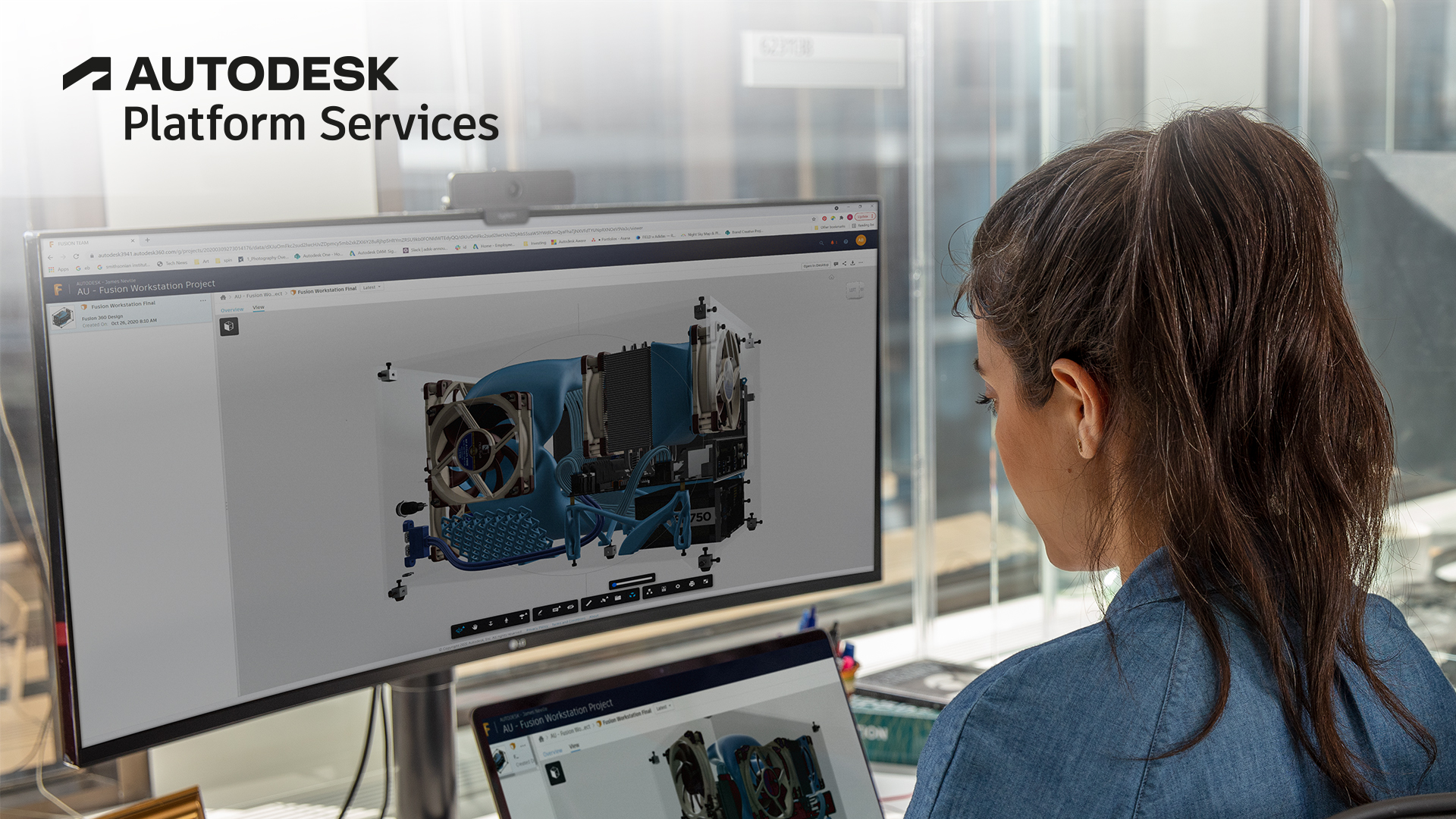
The new .NET SDK for Autodesk Platform Services have been out of beta for a couple of months already. Let's take a look at how you could migrate your .NET applications to this new official SDK.
Packaging & Installation
The new SDK is split into separate NuGet packages for individual APIs, for example:
Moreover, the SDK includes a "manager" module - Autodesk.SDKManager - that provides shared functionality and configuration (e.g., authentication, resiliency, or logging) for all the individual API modules.
Tip: you can see all modules available as part of this SDK on https://www.nuget.org/profiles/AutodeskPlatformServices.SDK.
When adding these dependencies to your project, simply install the SDK manager and the APIs you need, either using the NuGet manager UI in Visual Studio, or using the command line:
dotnet add package Autodesk.SdkManager
dotnet add package Autodesk.Authentication
dotnet add package Autodesk.OSS
dotnet add package Autodesk.ModelDerivative
Initialization
Each API module exposes a *Client
class - for example, AuthenticationClient
or OssClient
- that will be your entrypoint to the corresponding APS service. Constructors of all these classes expect an instance of the SDK manager as their only parameter. Here's an example of how these clients could be initialized:
using Autodesk.SDKManager;
using Autodesk.Authentication;
using Autodesk.Oss;
using Autodesk.ModelDerivative;
// ...
var sdkManager = SdkManagerBuilder.Create().Build();
var authenticationClient = new AuthenticationClient(sdkManager);
var ossClient = new OssClient(sdkManager);
var modelDerivativeClient = new ModelDerivativeClient(sdkManager);
Authentication & User Info
Generating 2-legged tokens:
using Autodesk.Authentication;
using Autodesk.Authentication.Model;
// ...
var scopes = new List<Scopes> { Scopes.DataRead, Scopes.ViewablesRead };
var auth = await authenticationClient.GetTwoLeggedTokenAsync(APS_CLIENT_ID, APS_CLIENT_SECRET, scopes);
Console.WriteLine(auth.AccessToken);
Generating 3-legged tokens:
using Autodesk.Authentication;
using Autodesk.Authentication.Model;
// ...
// Generating the authorization URL
var url = authenticationClient.Authorize(APS_CLIENT_ID, ResponseType.Code, APS_CALLBACK_URL, scopes);
Console.WriteLine(url);
// Exchanging temporary code for an access token
var threeLeggedCredentials = await authenticationClient.GetThreeLeggedTokenAsync(APS_CLIENT_ID, APS_CLIENT_SECRET, temporaryCode, APS_CALLBACK_URL);
Console.WriteLine(threeLeggedCredentials.AccessToken);
// Refreshing an access token
var refreshedCredentials = await authenticationClient.GetRefreshTokenAsync(APS_CLIENT_ID, APS_CLIENT_SECRET, threeLeggedCredentials.RefreshToken, scopes);
Console.WriteLine(refreshedCredentials.AccessToken);
Finally, here's how you would extract user information from an existing 3-legged token:
var userInfo = await authenticationClient.GetUserInfoAsync(threeLeggedCredentials.AccessToken);
Console.WriteLine(userInfo.Name);
Note: in v2 of the Authentication API, the
firstName
andlastName
fields have been replaced by a single field calledname
.
Convenience Methods
In certain cases the API clients may expose convenience methods that combine two or more underlying endpoint calls into a single method call. For example, when uploading files to an OSS bucket, instead of creating an upload URL, manually uploading the content, and finally sending a request to complete the upload, you can simply call:
await ossClient.Upload(bucketKey, objectName, sourceToUpload, accessToken, CancellationToken.None);
We're currently setting up a documentation and a changelog for the SDK project, and we'll highlight these options there.
Additional Resources
Before the official documentation for the new SDKs is ready, you can learn more about its usage by checking out the samples (part of the project's GitHub repository: https://github.com/autodesk-platform-services/aps-sdk-net), or our tutorials: https://tutorials.autodesk.io.