28 Aug 2023
Loading models in parallel
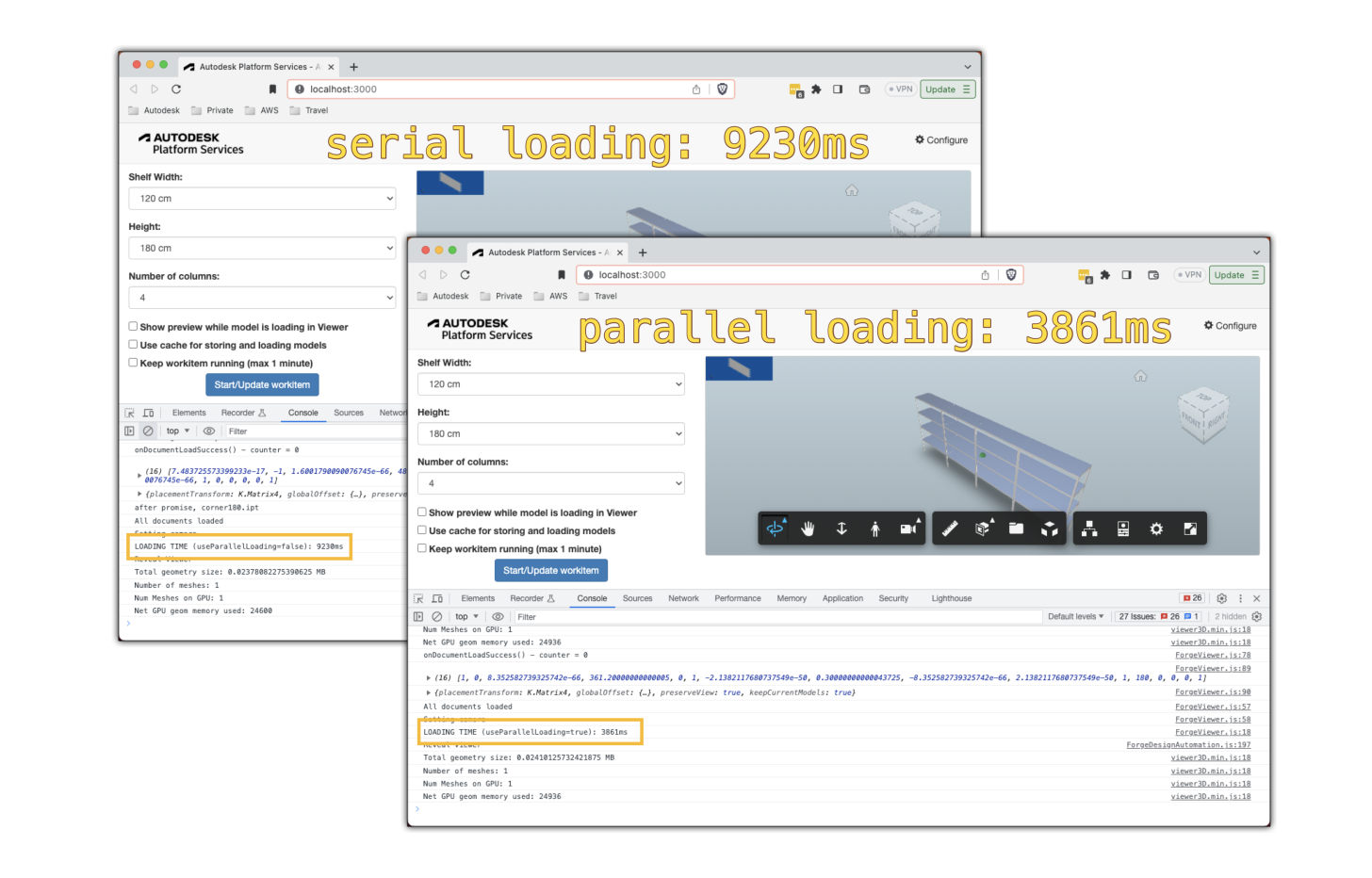
There are some blog posts on loading multiple models into the viewer (e.g. this one), but they don't seem to mention that you can do that in parallel as well: i.e. you don't have to wait for the first model to load before starting loading the next one.
In order to test the speed benefits of this, I added such an option to my https://inventor-configurator.autodesk.io/ sample (source code). It will load the models in parallel if window.useParallelLoading is set to true.
When using the option with most components, the full model loaded twice as fast - see picture on top:
function loadModels(data) {
return new Promise(async (resolve) => {
console.log('loadModels()');
let promises = [];
for (key in data.components) {
let component = data.components[key]
var objectName = data.urnBase + component.fileName
var documentId = 'urn:' + btoa(objectName);
console.log('before promise, ' + component.fileName)
window.useParallelLoading ?
promises.push(loadModel(documentId, component)) :
await loadModel(documentId, component);
console.log('after promise, ' + component.fileName)
}
if (window.useParallelLoading) {
await Promise.allSettled(promises);
}
console.log('All documents loaded');
console.log("Setting camera")
// Doing this instead of "viewer.autocam.cube.cubeRotateTo('front top right')"
// because that does it as a transition, i.e. slow
viewer.navigation.setWorldUpVector(new THREE.Vector3(0, 0, 1))
let pos = new THREE.Vector3(1,-1,1)
let target = new THREE.Vector3(0,0,0)
viewer.navigation.setView (pos, target)
let upVector = new THREE.Vector3(0,0,1)
viewer.navigation.setCameraUpVector (upVector)
viewer.utilities.fitToView(true)
resolve()
})
}