23 Feb 2019
How to generate dynamic number of output with Design Automation for Revit V3
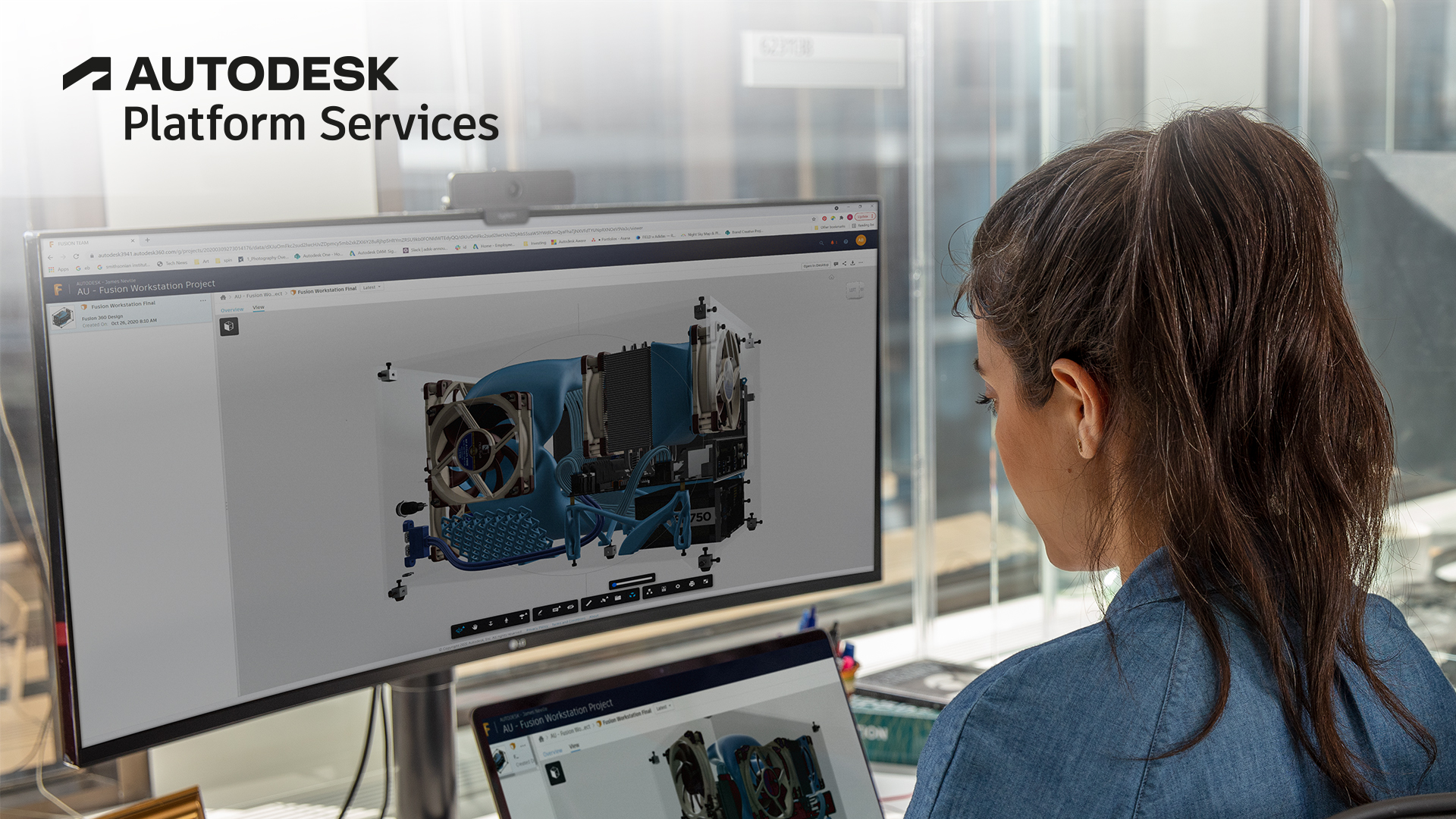
With the public beta of Design Automation for Revit V3 API announced, we have many partners who are interested in this service, and we recently hear of one question about how to generate dynamic number of result. For example, there is a request to export all the 2D sheets from one Revit model, let’s use this as an example to see how to implement.
The solution is simple, the key is to define an activity including the output parameter “resultrvt” with “zip=true” as follow:
{
"id": "ExportToDwgsActivity",
"commandLine": [ "$(engine.path)\\\\revitcoreconsole.exe /i $(args[rvtFile].path) /al $(appbundles[ExportToDwgsApp].path)" ],
"parameters": {
"rvtFile": {
"zip": false,
"ondemand": false,
"verb": "get",
"description": "Input Revit model",
"required": true
},
"resultrvt": {
"zip": true,
"ondemand": false,
"verb": "put",
"description": "Exported Dwg files",
"required": true,
"localName": "exportedDwgs"
}
},
"engine": "Autodesk.Revit+2019",
"appbundles": [ "{{dasNickName}}.ExportToDwgsApp+dev" ],
"description": "Export 2D sheet from Revit model to Dwgs."
}
What does the “zip=true” actually mean? For the zipped input file, it's well documented at https://forge.autodesk.com/en/docs/design-automation/v3/tutorials/revit/step6-post-workitem/, but for the output zipped result, it's not so clear, the key parameter here you need to understand is localName as shown above. Actually, when the workitem is executed, instead of uploading a normal result file to the specified output Url, Design Automation for Revit engine will find the subfolder with the name of exportedDwgs, and zip package it into exportedDwgs.zip, then upload the file to the specified output Url. What your plugin need to do is just export all the files under the folder of exportedDwgs, for this sample, the code should be like:
protected void RevitExportToDwgs( DesignAutomationData data )
{
if (data == null)
throw new ArgumentNullException(nameof(data));
Application rvtApp = data.RevitApp;
if (rvtApp == null)
throw new InvalidDataException(nameof(rvtApp));
string modelPath = data.FilePath;
if (String.IsNullOrWhiteSpace(modelPath))
throw new InvalidDataException(nameof(modelPath));
Document doc = data.RevitDoc;
if (doc == null)
throw new InvalidOperationException("Could not open document.");
using (Autodesk.Revit.DB.Transaction trans = new Transaction(doc, "ExportToDwgs"))
{
try
{
trans.Start();
List<View> views = new FilteredElementCollector(doc)
.OfClass(typeof(View))
.Cast<View>()
.Where(vw =>
vw.ViewType == ViewType.DrawingSheet && !vw.IsTemplate
).ToList();
List<ElementId> viewIds = new List<ElementId>();
foreach (View view in views)
{
ViewSheet viewSheet = view as ViewSheet;
viewIds.Add(viewSheet.Id);
}
DWGExportOptions options = new DWGExportOptions();
ExportDWGSettings dwgSettings = ExportDWGSettings.Create(doc, "mySetting");
options = dwgSettings.GetDWGExportOptions();
options.MergedViews = true;
if (RuntimeValue.RunOnCloud)
{
doc.Export( Directory.GetCurrentDirectory()+ "\\exportedDwgs", "rvt", viewIds, options);
}
else
{
// For local test
doc.Export(Path.GetDirectoryName(modelPath)+ "\\exportedDwgs", "rvt", viewIds, options);
}
trans.Commit();
}
catch (Exception ee)
{
System.Diagnostics.Debug.WriteLine(ee.Message);
System.Diagnostics.Debug.WriteLine(ee.StackTrace);
return;
}
finally
{
if (trans.HasStarted())
trans.RollBack();
}
}
}
Now we can post a workitem to execute the activity with the payload as follow, and after the workitem is successfully completed, you should get the zipped file within the signed Url "{{exportToDwgsResultSignedUrl}}" that is specified in your workitem, and continue the operations to the zipped result per your request.
{
"activityId":"{{dasNickName}}.ExportToDwgsActivity+dev",
"arguments":{
"rvtFile":{
"url":"https://developer.api.autodesk.com/oss/v2/buckets/{{bucketKey}}/objects/revitModel.rvt",
"Headers":{
"Authorization":"Bearer {{dataApiToken}}"
}
},
"resultrvt":{
"verb":"put",
"url":"{{exportToDwgsResultSignedUrl}}"
}
}
}