25 Feb 2020
Dealing with suppressed components
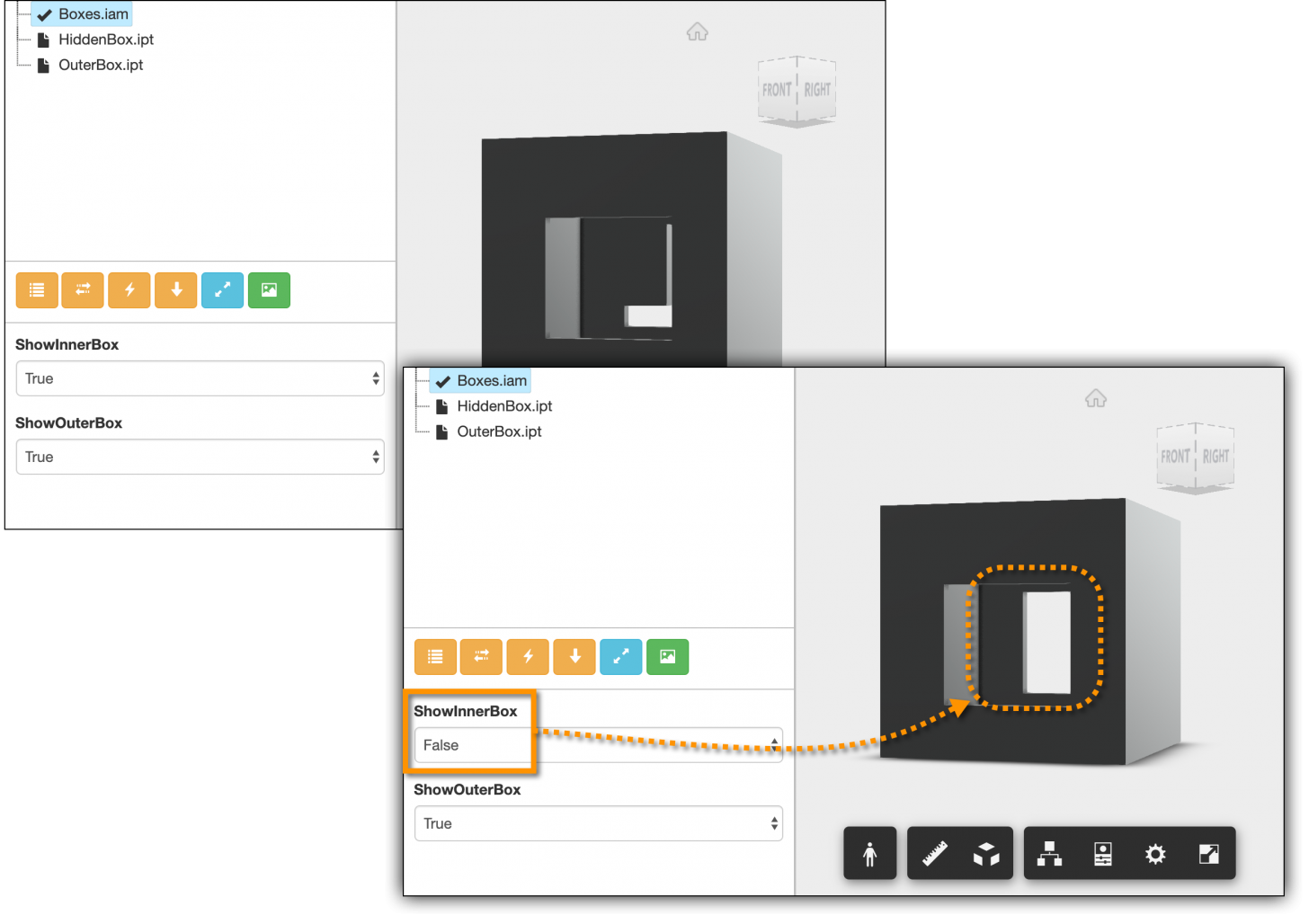
In Inventor one way to hide a component is by suppressing it. Unlike when simply making it invisible, in this case the relevant document won't even get loaded, so it's more efficient.
When opening an assembly programmatically, by default the Master LOD (the main Level Of Detail) will be loaded which has all components available/unsuppressed.
However, information about the LOD that was last active when the document got saved is stored in the document, and we can use that to open that specific LOD:
Document doc = null;
if (docPath.ToUpper().EndsWith(".IAM"))
{
FileManager fm = inventorApplication.FileManager;
string dvActRep = fm.GetLastActiveDesignViewRepresentation(docPath);
LogTrace($"LastActiveDesignViewRepresentation: {dvActRep}");
string lodActRep = fm.GetLastActiveLevelOfDetailRepresentation(docPath);
LogTrace($"LastActiveLevelOfDetailRepresentation: {lodActRep}");
NameValueMap openOptions = inventorApplication.TransientObjects.CreateNameValueMap();
openOptions.Add("LevelOfDetailRepresentation", lodActRep);
openOptions.Add("DesignViewRepresentation", dvActRep);
doc = inventorApplication.Documents.OpenWithOptions(docPath, openOptions, false);
}
else
{
doc = inventorApplication.Documents.Open(docPath, false);
}
LogTrace($"Full document name: {doc.FullDocumentName}");
Opening the assembly visible seems to cause problems, at least when it comes to suppressed components:
- trying to set a component suppressed will end the process ?
- suppressed components will be visible in the SVF (the format used by the Forge Viewer) generated by Inventor
It's a good practice in general to open the document invisible when using Automation, so just set the last parameter of Open/OpenWithOptions function called OpenVisible to false - just like in the above code.
One note concerning the Model Derivative API: currently it uses the Master LOD of the assembly, so suppressed components will be visible in the SVF file
In case of other scenarios, it's also useful to activate the relevant Inventor project file (*.ipj) before opening the assembly, as it sometimes may be necessary for file resolution. In this case I had the path to the project file passed to my WorkItem in a parameters file called inputParams.json:
Dictionary<string, string> options =
JsonConvert.DeserializeObject<Dictionary<string, string>>(
System.IO.File.ReadAllText("inputParams.json"));
if (options.ContainsKey("projectFile"))
{
string projectFile = options["projectFile"];
string fullProjectPath = Path.GetFullPath(Path.Combine(currDir, projectFile));
Console.WriteLine("fullProjectPath = " + fullProjectPath);
DesignProject dp = inventorApplication.DesignProjectManager.DesignProjects.AddExisting(fullProjectPath);
dp.Activate();
}
In my test assembly I had two boolean User Parameters (ShowInnerBox and ShowOuterBox) and they were driving which components got suppressed through this simple iLogic rule:
Dim compInner = Component.InventorComponent("HiddenBox:1")
Dim compOuter = Component.InventorComponent("OuterBox:1")
If ShowInnerBox Then
If compInner.Suppressed Then
compInner.Unsuppress()
End If
Else
If Not compInner.Suppressed Then
compInner.Suppress(True)
End If
End If
If ShowOuterBox Then
If compOuter.Suppressed Then
compOuter.Unsuppress()
End If
Else
If Not compOuter.Suppressed Then
compOuter.Suppress(True)
End If
End If
iLogicVb.UpdateWhenDone = True
You can see the result in the above picture.